Java 8 findFirst() vs findAny()
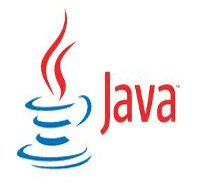
In this tutorial, I am showing the differences between findFirst() and findAny() methods of java 8 stream api and where it can be used.
Note: As per the stream documentation “Streams may or may not have a defined encounter order. Whether or not a stream has an encounter order depends on the source and the intermediate operations. Certain stream sources (such as List or arrays) are intrinsically ordered, whereas others (such as HashSet) are not”.
findAny()
findAny() method returns any element from a stream, there is no guarantee about the order of the returned element. If you check below output the program returns Optional[A] but it can be returned Optional[B] or Optional[C] in another execution.
package com.javatips; import java.util.Optional; import java.util.stream.Stream; public class StreamFindAny { public static void main(String[] args) { Stream<String> stream = Stream.of("A","B","C"); Optional<String> result = stream.findAny(); System.out.println(result); } }
Output
Optional[A]
findFirst()
findFirst() method returns first element from a stream. If you check below output the program returns Optional[A]
package com.javatips; import java.util.Optional; import java.util.stream.Stream; public class StreamFindFirst { public static void main(String[] args) { Stream<String> stream = Stream.of("A","B","C"); Optional<String> result = stream.findFirst(); System.out.println(result); } }
Output
Optional[A]