Evaluate/Check List/Collection Is Empty In JSTL
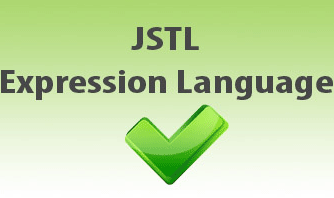
Evaluate/Check List/Collection Is Empty In JSTL explains about How to evaluate whether a List or Collection is empty using JSTL empty operator.
Consider the example here, a JSP page which contains a HashMap with lot of key value pairs in it and we need check this list is empty or not
For evaluating a Collection is empty or not inside JSTL, we can use empty operator. By using this operator we can check Collection is empty or not
JSTL Size Of Map
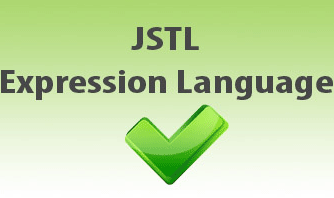
JSTL Size Of Map explains about how to find the size of a map using JSTL Taglib
Consider the example here, a JSP page which contains a HashMap with lot of key value pairs in it and we need to find the size of this HashMap
We already seen how to find the size of a Collection using the JSTL fn:length function.
You can see it fn:length() JSTL Function .
JSTL If Else Example
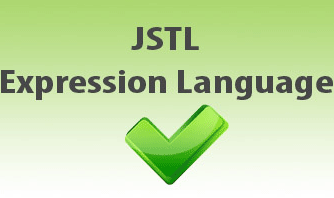
JSTL If Else Example explains about testing different conditions as per the requirements. It also provides a secondary path (else case) of execution, when an "if" conditions become false.
We have already seen the usage of JSTL If Example
How to use if-else option in JSTL?
There are 2 ways, we can achieve if-else statements using JSTL
1) You can use JSTL C:Choose C:When C:Otherwise Tag
2) You can use JSTL Mod (modulus) operator
You can see the below example, which is demonstrating JSTL If Else Example Using Mod operator
JSTL c:url Example With c:param Tag
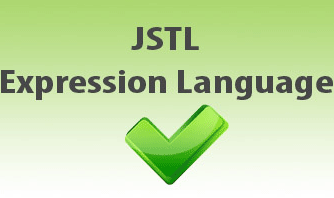
JSTL <c:url> tag is used for storing a url into a variable with proper url rewriting, mostly <c:url> is used with <c:param> tag for adding parameter inside a <c:url>.
You can see required attributes for <c:url> and <c:param> tags on the below example.
<c:url> With Request scope
<c:url var="url" value="/index.jsp" context="/JSTLExample" scope="request"/>
${requestScope.url}
<c:url> With Session scope
<c:url var="url" value="/index.jsp" context="/JSTLExample" scope="session"/>
${sessionScope.url}
Get context Path Using JSTL
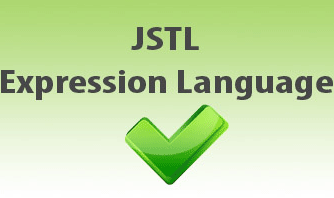
Get context Path Using JSTL explains about how to get context path inside a JSP page
pageContext is an implicit object available in JSP, so it is very easy to get the contextPath using JSTL EL Expression
You can access the request scoped varaibles in following different ways
Below code is equivalent to <%=request.getContextPath()%> in JSTL?
<c:out value="${pageContext.request.contextPath}"/>
you can see the below a complete example about accessing contextpath in JSTL
Reading Request Attributes Using JSTL
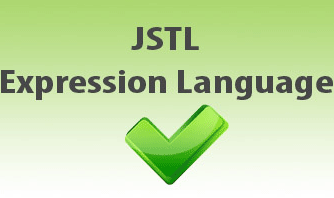
Reading Request Attributes Using JSTL explains about how to access JSTL's request scoped attributes such as variables inside a JSP page
request is an implicit object available in JSP, so it is very easy to access the request scoped varaibles inside JSP page
You can access the request scoped varaibles in following different ways
<c:out value='${requestScope["variableName"]}'/>
<c:out value='${requestScope.variableName}'/>
you can see the below example demonstrating how to access request scoped attributes in JSTL EL expression
Reading Session Attributes Using JSTL
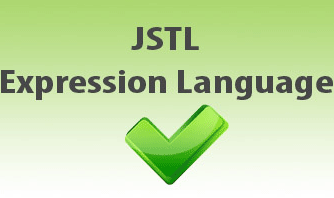
Reading Session Attributes Using JSTL explains about how to access JSTL's session scoped attributes such as variables inside a JSP page
session is an implicit object available in JSP, so it is very easy to access the session scoped varaibles inside JSP page
You can access the session scoped varaibles in following different ways
<c:out value='${sessionScope["variableName"]}'/>
<c:out value='${sessionScope.variableName}'/>
you can see the below complete example demonstrating how to access session scoped attributes in JSTL EL expression
JSTL forTokens Example
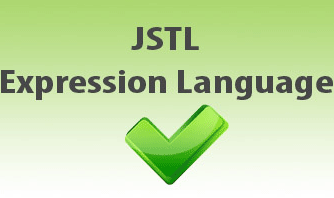
JSTL forTokens Example explains about how to use JSTL forTokens inside JSP and its various mandatory and optional attributes.
c:forTokens Tag is used for splitting a string into tokens and iterate through each of the tokens, c:forTokens Tag is available as a part of JSTL core tags
Inside c:forTokens Tag, you need to specify following mandatory attributes.
items -> These values (token strings) are used to iterate with delimiters.
delims -> These values are that split / separate the token string
Inside c:forTokens Tag, you need to specify following optional attributes.
begin -> Starting element from
end -> Ending element
step -> Iterating steps
var -> Current item
varStatus -> Name of the variable inside loop
You can see the below example demonstrating the usage JSTL c:forTokens Tag Example inside JSTL