CXF Tutorial With Apache Maven
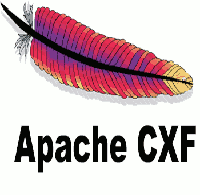
In this tutorial, we are implementing a jax-ws web service using Apache CXF with Maven and deployed in Tomcat.
Apache CXF is a free and open source project, and a fully featured Web service framework. It helps you building web services using different front-end API's, like as JAX-RS and JAX-WS.
Services will talk different protocols such as SOAP, RESTful HTTP, CORBA & XML/HTTP and work with different transports like JMS, HTTP or JBI.
pom.xml
You need to have following dependencies
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.javatips.net</groupId> <artifactId>cxftutorial</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> <name>cxftutorial Maven Webapp</name> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> </properties> <dependencies> <dependency> <groupId>org.apache.cxf</groupId> <artifactId>cxf-rt-frontend-jaxws</artifactId> <version>3.3.6</version> </dependency> <dependency> <groupId>org.apache.cxf</groupId> <artifactId>cxf-rt-transports-http</artifactId> <version>3.3.6</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-web</artifactId> <version>5.2.6.RELEASE</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>5.2.6.RELEASE</version> </dependency> </dependencies> </project>
Create a Student Object
package com.student; public class Student { private String name; public String getName() { return name; } public void setName(String name) { this.name = name; } }
Create a Service Interface
This service interface will defines which methods of web service, to be invoked by the client
package com.student; import javax.jws.WebService; @WebService public interface ChangeStudentDetails { Student changeName(Student student); }
Implement the Service Interface
Here we implement the service interface created on the previous step
package com.student; import javax.jws.WebService; @WebService(endpointInterface = "com.student.ChangeStudentDetails") public class ChangeStudentDetailsImpl implements ChangeStudentDetails { public Student changeName(Student student) { student.setName("Hello "+student.getName()); return student; } }
Create a cxf.xml
CXF is using Spring internally, Finding classes by spring we need to add service implementation class on "jaxws:endpoint" tag
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:jaxws="http://cxf.apache.org/jaxws" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://cxf.apache.org/jaxws http://cxf.apache.org/schemas/jaxws.xsd"> <jaxws:endpoint id="changeStudent" implementor="com.student.ChangeStudentDetailsImpl" address="/ChangeStudent" /> </beans>
web.xml
Modify web.xml file to find CXF servlet and cxf.xml
<!DOCTYPE web-app PUBLIC "-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN" "http://java.sun.com/dtd/web-app_2_3.dtd" > <web-app> <display-name>Archetype Created Web Application</display-name> <context-param> <param-name>contextConfigLocation</param-name> <param-value>WEB-INF/cxf.xml</param-value> </context-param> <listener> <listener-class>org.springframework.web.context.ContextLoaderListener</listener-class> </listener> <servlet> <servlet-name>CXFServlet</servlet-name> <servlet-class>org.apache.cxf.transport.servlet.CXFServlet</servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>CXFServlet</servlet-name> <url-pattern>/*</url-pattern> </servlet-mapping> </web-app>
Publishing CXF Web Service
Deployed CXF Web Service