Getting IP Address Using CXF
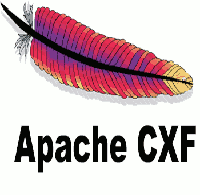
Getting IP Address Using CXF explains about How to get IP Address of client who is consuming the CXF service
I am showing here, How to capture the remote IP Address of clients, both SOAP & RESTFul services
Message message = PhaseInterceptorChain.getCurrentMessage(); HttpServletRequest request = (HttpServletRequest)message.get(AbstractHTTPDestination.HTTP_REQUEST); request.getRemoteAddr()
On this code, we are getting HttpServletRequest and from that taking the remote ip address
You can see the below example, which is finding client ip address using cxf
Getting IP Address Using CXF
I am going to re-use CXF Restful Tutorial
I just added a new property "studentIP" into student object. please find the code below
package com.student;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement(name = "Student")
public class Student {
private String name;
private String studentIP;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getStudentIP() {
return studentIP;
}
public void setStudentIP(String studentIP) {
this.studentIP = studentIP;
}
}
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement(name = "Student")
public class Student {
private String name;
private String studentIP;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getStudentIP() {
return studentIP;
}
public void setStudentIP(String studentIP) {
this.studentIP = studentIP;
}
}
We are making changes ChangeStudentDetailsImpl class, just adding
PhaseInterceptorChain.getCurrentMessage() and getting HttpServletRequest, so that we can access remote ip of clients consuming our service
package com.student;
import javax.servlet.http.HttpServletRequest;
import javax.ws.rs.Consumes;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import org.apache.cxf.message.Message;
import org.apache.cxf.phase.PhaseInterceptorChain;
import org.apache.cxf.transport.http.AbstractHTTPDestination;
@Consumes("application/json")
@Produces("application/json")
public class ChangeStudentDetailsImpl implements ChangeStudentDetails {
@POST
@Path("/changeName")
public Student changeName(Student student) {
student.setName("HELLO " + student.getName());
// Here We Capture/Extracting Client IP Address Using CXF
Message message = PhaseInterceptorChain.getCurrentMessage();
HttpServletRequest request = (HttpServletRequest)message.get(AbstractHTTPDestination.HTTP_REQUEST);
student.setStudentIP(request.getRemoteAddr());
return student;
}
@GET
@Path("/getName")
public Student getName() {
Student student = new Student();
student.setName("Rockey");
return student;
}
}
import javax.servlet.http.HttpServletRequest;
import javax.ws.rs.Consumes;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import org.apache.cxf.message.Message;
import org.apache.cxf.phase.PhaseInterceptorChain;
import org.apache.cxf.transport.http.AbstractHTTPDestination;
@Consumes("application/json")
@Produces("application/json")
public class ChangeStudentDetailsImpl implements ChangeStudentDetails {
@POST
@Path("/changeName")
public Student changeName(Student student) {
student.setName("HELLO " + student.getName());
// Here We Capture/Extracting Client IP Address Using CXF
Message message = PhaseInterceptorChain.getCurrentMessage();
HttpServletRequest request = (HttpServletRequest)message.get(AbstractHTTPDestination.HTTP_REQUEST);
student.setStudentIP(request.getRemoteAddr());
return student;
}
@GET
@Path("/getName")
public Student getName() {
Student student = new Student();
student.setName("Rockey");
return student;
}
}
Run Client
package com.client;
import java.io.IOException;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Scanner;
// Getting The IP Address Of SOAP Clients Using CXF
public class PostStudentClient {
public static void main(String[] args) {
try {
URL url = new URL("http://localhost:8080/CXFRestfulTutorial/rest/changeName");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(true);
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/json");
String input = "{\"Student\":{\"name\":\"Tom\"}}";
OutputStream os = conn.getOutputStream();
os.write(input.getBytes());
os.flush();
Scanner scanner;
String response;
if (conn.getResponseCode() != 200) {
scanner = new Scanner(conn.getErrorStream());
response = "Error From Server \n\n";
} else {
scanner = new Scanner(conn.getInputStream());
response = "Response From Server \n\n";
}
scanner.useDelimiter("\\Z");
System.out.println(response + scanner.next());
scanner.close();
conn.disconnect();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
import java.io.IOException;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Scanner;
// Getting The IP Address Of SOAP Clients Using CXF
public class PostStudentClient {
public static void main(String[] args) {
try {
URL url = new URL("http://localhost:8080/CXFRestfulTutorial/rest/changeName");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(true);
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/json");
String input = "{\"Student\":{\"name\":\"Tom\"}}";
OutputStream os = conn.getOutputStream();
os.write(input.getBytes());
os.flush();
Scanner scanner;
String response;
if (conn.getResponseCode() != 200) {
scanner = new Scanner(conn.getErrorStream());
response = "Error From Server \n\n";
} else {
scanner = new Scanner(conn.getInputStream());
response = "Response From Server \n\n";
}
scanner.useDelimiter("\\Z");
System.out.println(response + scanner.next());
scanner.close();
conn.disconnect();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output
Response From Server {"Student":{"name":"HELLO Tom","studentIP":"117.196.145.171"}}
6 Responses to "Getting IP Address Using CXF"