Change CXF Character Encoding
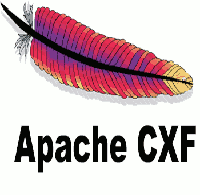
Change CXF Character Encoding explains about How to change character encoding of CXF based messages
Usually CXF not change the encoding of messages, but you can deliberately change one encoding to another by specifying a particular charset that we needed.
Here I am showing an CXF Character Encoding Example where you can see How to change one encoding to another, covering both JAX-WS & JAX-RS services
Change Character Encoding In CXF Restful Services
I am going to re-use CXF Restful Tutorial
We are making changes ChangeStudentDetailsImpl class, just adding
@Produces("application/json; charset=UTF-8")
import javax.ws.rs.Consumes;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
@Consumes("application/json")
@Produces("application/json; charset=UTF-8")
public class ChangeStudentDetailsImpl implements ChangeStudentDetails {
@POST
@Path("/changeName")
public Student changeName(Student student) {
student.setName("HELLO " + student.getName());
return student;
}
@GET
@Path("/getName")
public Student getName() {
Student student = new Student();
student.setName("Rockey");
return student;
}
}
Run CXF REST Client
import java.io.IOException;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Scanner;
//CXF REST/JAX-RS Encoding Example
public class PostStudentClient {
public static void main(String[] args) {
try {
URL url = new URL("http://localhost:8082/CXFRestfulTutorial/rest/changeName");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(true);
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/json;charset=ISO-8859-1");
String input = "{\"Student\":{\"name\":\"Tom\"}}";
OutputStream os = conn.getOutputStream();
os.write(input.getBytes());
os.flush();
Scanner scanner;
String response;
if (conn.getResponseCode() != 200) {
scanner = new Scanner(conn.getErrorStream());
response = "Error From Server \n\n";
} else {
System.out.println(conn.getContentType());
scanner = new Scanner(conn.getInputStream());
response = "Response From Server \n\n";
}
scanner.useDelimiter("\\Z");
System.out.println(response + scanner.next());
scanner.close();
conn.disconnect();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output
From the output screenshot you can see that even we are setting charset as ISO-8859-1 in client side by using the below code
conn.setRequestProperty("Content-Type", "application/json;charset=ISO-8859-1");
Response payload producing UTF-8 charset
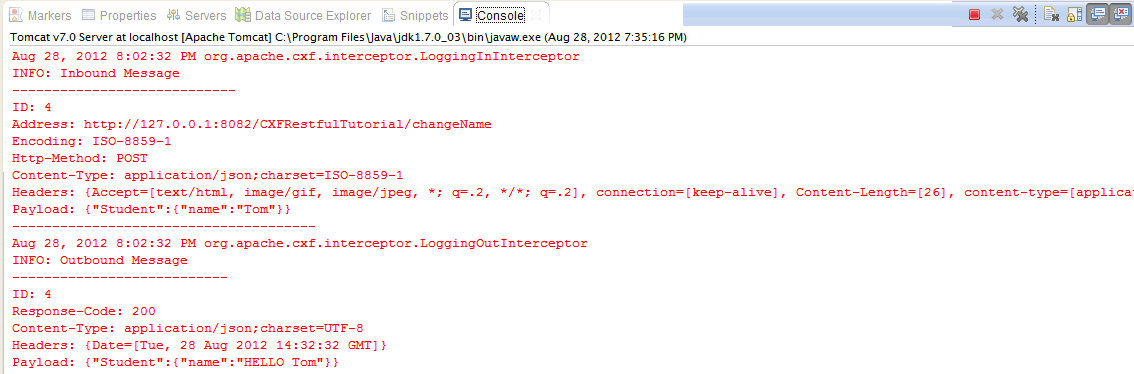
Change Character Encoding In CXF JAX-WS Services
I am going to re-use CXF Web Service Tutorial
We are making changes ChangeStudentDetailsImpl class, just adding
Message message = PhaseInterceptorChain.getCurrentMessage();
message.put(Message.ENCODING, "ASCII");
import javax.jws.WebService;
import org.apache.cxf.message.Message;
import org.apache.cxf.phase.PhaseInterceptorChain;
@WebService(endpointInterface = "com.student.ChangeStudentDetails")
public class ChangeStudentDetailsImpl implements ChangeStudentDetails {
public Student changeName(Student student) {
student.setName("Hello " + student.getName());
Message message = PhaseInterceptorChain.getCurrentMessage();
message.put(Message.ENCODING, "ASCII");
return student;
}
}
Run CXF SOAP Client
import org.apache.cxf.interceptor.LoggingInInterceptor;
import org.apache.cxf.interceptor.LoggingOutInterceptor;
import org.apache.cxf.jaxws.JaxWsProxyFactoryBean;
import com.student.ChangeStudentDetails;
import com.student.Student;
//CXF JAX-WS Encoding Example
public final class StudentClient {
public static void main(String args[]) throws Exception {
JaxWsProxyFactoryBean factory = new JaxWsProxyFactoryBean();
factory.setServiceClass(ChangeStudentDetails.class);
factory.setAddress("http://localhost:8080/CXFTutorial/ChangeStudent?wsdl");
factory.getInInterceptors().add(new LoggingInInterceptor());
factory.getOutInterceptors().add(new LoggingOutInterceptor());
ChangeStudentDetails client = (ChangeStudentDetails) factory.create();
Student student = new Student();
student.setName("Rockey");
Student changeName = client.changeName(student);
System.out.println("Server said: " + changeName.getName());
System.exit(0);
}
}
Output
From the output screenshot you can see that Response payload producing ASCII charset
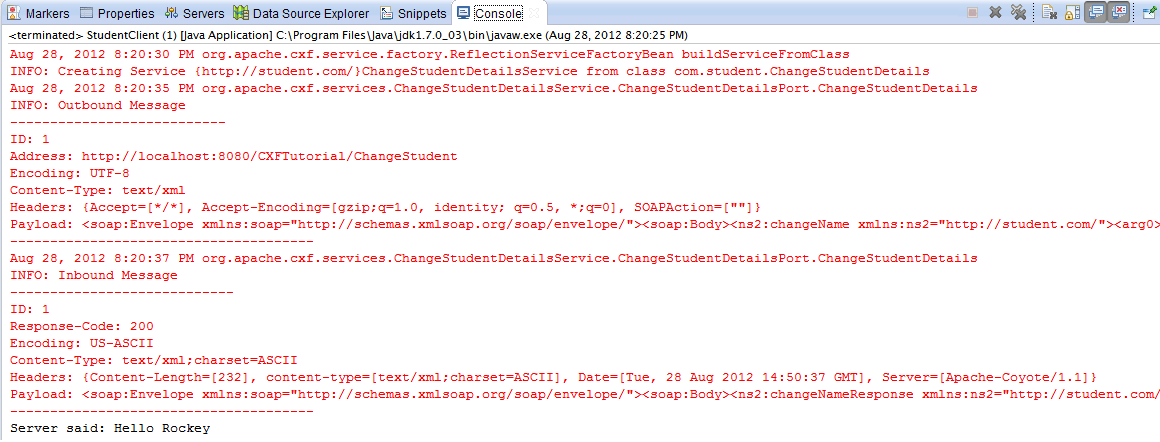