Spring Tutorial
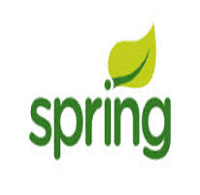
Spring Tutorial explains step by step details of setting / configuring Spring with Eclipse
The Spring Framework is a free and open source framework using for the development of java enterprise applications
Spring framework consists of several different modules including Aspect Oriented Programming, Inversion Control, Model View Controller, Data Access, Transaction Management, Batch Processing, Remote Access & Spring JDBC Template will really ease the development effort of coding team.
In this example you will learn how to set / injects a bean property via setter injection using spring framework.
Required Libraries
You need to download
Following jar must be in classpath
- org.springframework.asm-3.0.6.RELEASE.jar
- org.springframework.beans-3.0.6.RELEASE.jar
- org.springframework.context-3.0.6.RELEASE.jar
- org.springframework.context.support-3.0.6.RELEASE.jar
- org.springframework.core-3.0.6.RELEASE.jar
- org.springframework.expression-3.0.6.RELEASE.jar
- antlr-2.7.7.jar
- commons-logging-1.1.1.jar
Create domain model
Create domain model named student. Here we are going to inject 2 properties via setter injection.
Consider the following Student bean class
public class Student {
private String name;
private int age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String toString() {
return "Student " + name + " is " + age + " years old";
}
}
beans.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <bean id="student" class="com.javatips.Student"> <property name="name" value="Rockey" /> <property name="age" value="29" /> </bean> </beans>
Test Program
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Main {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("beans.xml");
Student student = (Student)context.getBean("student");
System.out.println(student);
}
}