Spring MVC 4 REST Example
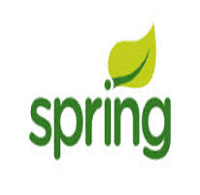
Spring MVC 4 REST Example explains about creating a simple Spring MVC REST service using eclipse.
In this example we are using Jackson JSON API for returning JSON response according to the request
Now a days more and more deployment is going based on restful services compare to WSDL Webservices, due to the weights towards the simplicity of configuration
You can see the below example, which is demonstrating How to create a Spring Restful service using MVC 4
Required Libraries
Following jar must be in classpath
- jackson-annotations-2.4.0.jar
- jackson-core-2.4.0.jar
- jackson-databind-2.4.0.jar
- commons-logging-1.1.1.jar
- spring-aop-4.0.5.RELEASE.jar
- spring-beans-4.0.5.RELEASE.jar
- spring-context-4.0.5.RELEASE.jar
- spring-core-4.0.5.RELEASE.jar
- spring-expression-4.0.5.RELEASE.jar
- spring-web-4.0.5.RELEASE.jar
- spring-webmvc-4.0.5.RELEASE.jar
You can see the project structure below
Student.java
public class Student {
private int studId;
private String name;
private int age;
public int getStudId() {
return studId;
}
public void setStudId(int studId) {
this.studId = studId;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
StudentController.java
In StudentController class, we have added @RestController annotation which removing the need to add @ResponseBody to each of your @RequestMapping methods.
@RequestMapping annotation is used for mapping web requests to corresponding handler classes and handler methods
import java.util.ArrayList;
import java.util.List;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
import com.student.domain.Student;
@RestController
@RequestMapping("/service/student/")
public class StudentController {
@RequestMapping(method = RequestMethod.GET, headers = "Accept=application/json")
public List<Student> getAllStudents() {
List<Student> Students = new ArrayList<Student>();
Student student = new Student();
student.setName("Rockey");
Students.add(student);
student = new Student();
student.setName("Jose");
Students.add(student);
return Students;
}
@RequestMapping(value = "/{id}", method = RequestMethod.GET, headers = "Accept=application/json")
public Student getStudentById(@PathVariable int id) {
Student student = null;
if (id == 1) {
student = new Student();
student.setName("Jose");
}else{
student = new Student();
student.setName("Invalid Student");
}
return student;
}
}
rest-servlet.xml
The name for rest-servlet.xml is must match the servlet-name we have provided on web.xml. In our case we have provided servlet-name as rest, so we need to append "-servlet.xml"
Below code is used for Spring auto detection feature of finding the packages where the controller is defined
<context:component-scan base-package="com.student.controller" />
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.0.xsd"> <context:component-scan base-package="com.student.controller" /> <mvc:annotation-driven /> </beans>
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>Spring MVC Rest</display-name> <servlet> <servlet-name>rest</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>rest</servlet-name> <url-pattern>/*</url-pattern> </servlet-mapping> </web-app>
Run