How to use Java File separator,separatorChar
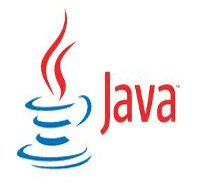
Directory separator using in Unix systems is forward slash (/) while in windows it is backward slash (\).
Due to this differences if we hard coded any of these slashes, it will not work on other environment and the program will not work in platform independent mode.
In Java we can use following different methods to achieve platform independent code
1) FileSystems.getDefault().getSeparator()
2) System.getProperty("file.separator")
3) File.separator
1. Java NIO - FileSystems.getDefault().getSeparator()
import java.nio.file.FileSystems; public class FileSeparatorExample { public static void main(String[] args) { String separator = FileSystems.getDefault().getSeparator(); System.out.println(separator); } }
output
/ (for unix) \ (for windows)
2. System.getProperty("file.separator")
public class FileSeparatorExample { public static void main(String[] args) { String separator = System.getProperty("file.separator"); System.out.println(separator); } }
output
/ (for unix) \ (for windows)
3. Java IO - File.separator
import java.io.File; public class FileSeparatorExample { public static void main(String[] args) { String separator = File.separator; System.out.println(separator); } }
output
/ (for unix) \ (for windows)
What is File.separatorChar
The value of separatorChar is same as File.Seperator, but it will be a char rather than a string. So the output for File.separatorChar is forward slash(/) for unix and backward slash (\) for windows.
File.separatorChar Example
import java.io.File; public class FileSeparatorExample { public static void main(String[] args) { System.out.println(File.separatorChar); } }
output
/ (for unix) \ (for windows)