How to Accept User Keyboard Input in Java
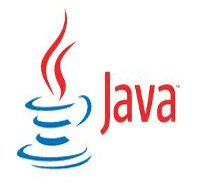
Java is not designed for hardware coding. But programmers do occasionally need to handle hardware issues with Java. It’s not uncommon for modern systems to require some form of user input, usually in the form of typing. This brief guide will explain how to code a Java program to accept user input from a keyboard.
Java Vs. Hardware
First, let’s look at why Java and hardware rarely mix. The main reason for this is that Java is designed to be platform independent. Hardware coding is highly device dependent. Java has the flexibility to adapt to any program, but if the program requires hardware compatibility, problems may arise.
Arguably, Java’s capabilities with hardware are limited. That doesn’t mean it’s not completely absent either. It’s possible to do minor hardware coding in Java and accept input from external devices as long as certain conditions are met. Therefore, before you begin, you need to know the type of operating system you will be using, the type of hardware involved, and whether data would be read-only or needs to be transmitted to the hardware device.
The input coding databases are in the Java Standard Edition (SE). The extra libraries in Java EE don’t have hardware input programs. Java has a mobile device support features in ME. However, what you need would be in Java SE.
Java User Input Coding Guide
Fortunately, developers are not required to write input codes from scratch. Java’s vast .util library should have the base for the code you need. Look for the Scanner class tools in the reference library. This is what will be used to code user input from a keyboard in a Java-based program.
Step 1
First, you need to import the Scanner class from the library. The code for this is rather simple: import java.util.Scanner;
Then you need to call up the class with “public class StringVariables {“ line. Now you have imported the Scanner class from the .util library.
Step 2
Now you need to create an object in this class. The class itself is just a unit of code. It’s useless on its own unless you give it an objective.
Create the object using this line:
Scanner user_input = new Scanner( System.in );
Step 3
Next, you need to set up a variable for the class. We are not creating an int or a String variable here. We are creating something new: a Scanner variable.
Set up the variable as user_input for convenience. Now we need to code that the object in this code line comes from a user typing. In other words, you need to tell the program that it’s System input. So put System.in inside the brackets to declare the object.
Step 4
The input now would be whatever the user types into the program. The Scanner object you just created has several methods available. We are setting up the next method as the text from the keyboard.
You can now create two String variables, or more, depending on the keyboard input. You need to use “ = user_input.next( );” to set up the variables. Use as many Strings as you like depending on the keyboard input.
The code should print to keep the cursor on the same line after input is received. If you use printIn, the cursor would move to a new line.
Step 5
Now you can execute the program. The final line of your code should have the print function to show the user input in the output window.
If you write your code properly, Java should execute the code only when the enter key is hit after user input. The program should wait for the user input with the go-ahead to execute coming from the enter key.
Getting the Code Right
The hardware code you program with Java would be simple, as mentioned above. But minor mistakes could cause problems even if everything were beginner level.
When you code for user input to a device, keep in mind that understanding the basic functions of the device is essential for getting your code right. You would need to know the application model as well.
All in all, Java is a great language to learn to execute simple hardware programming codes. You will be limited to what’s available in the reference library. However, you can stretch the limits of Java with simple codes like the user input one mentioned above.
Author Bio:
Ollie Mercer is an independent blogger who covers hardware and software issues. He studied coding as an undergraduate and was a devout tech employee for years before deciding to go freelance. He splits his time between California and New York, his home state.