Find Last Element Of A Stream
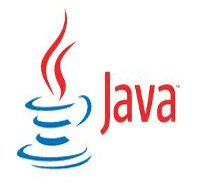
In this tutorial, here I am showing how to find the last element from a stream in java.
Note: we are using stream.reduce function for finding the last element of a stream in java. Stream.reduce method always returns Optional value, so you have to decide what to do when empty element is returned.
Find Last element of a Stream
The best way to get the last element of a stream is you can use stream().reduce method and below example you can see different versions of the method.
In case 1 you can see ‘D’ is returned and it is the last element of the stream. In case 2 there is no element in the stream and default element ‘E’ is returned. In case 3 there is no element in the stream and it is throwing an exception.
package com.javatips; import java.util.Arrays; import java.util.List; public class GetStreamLastElment { public static void main(String[] args) { // case 1 List<String> list = Arrays.asList("A", "B", "C", "D"); list.stream().reduce((a, b) -> b).ifPresent(s -> System.out.println(s)); // case 2 list = Arrays.asList(); System.out.println(list.stream().reduce((a, b) -> b).orElse("E")); // case 3 list.stream().reduce((a, b) -> b).orElseThrow(() -> new IllegalStateException("no last element")); } }
Output
D E Exception in thread "main" java.lang.IllegalStateException: no last element at com.javatips.GetStreamLastElment.lambda$4(GetStreamLastElment.java:16) at java.util.Optional.orElseThrow(Optional.java:290) at com.javatips.GetStreamLastElment.main(GetStreamLastElment.java:16)