Send Email Using Java
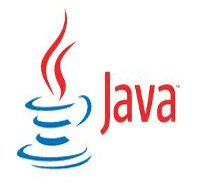
In this tutorial, I am showing how to send an email using JavaMail Api. Sending email is a required use case for every project.
Here I am using gmail host( smtp.gmail.com) and gmail username and password for authentication. For a bigger projects, you have to use paid mail service provider and they will provide the required information like host and port parameters.
Here I am explaining following topics with complete examples
a) Sending plain text Email with JavaMail API
b) Sending HTML email with JavaMail API
c) Sending email attachment with JavaMail API
Dependency
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.javatips</groupId> <artifactId>javatips.net</artifactId> <version>0.0.1-SNAPSHOT</version> <dependencies> <dependency> <groupId>javax.mail</groupId> <artifactId>mail</artifactId> <version>1.5.0-b01</version> </dependency> </dependencies> </project>
a) Sending Plain text Email with JavaMail API
package com.javatips; import java.util.*; import javax.mail.*; import javax.mail.internet.*; public class SendEmail { public static void main(String[] args) { String from = "from@gmail.com"; String pass = "password"; String[] toAddress = new String[]{"to@gmail.com"}; String subject = "Java Mail Example"; String body = "Hi, how are you"; Properties properties = System.getProperties(); String host = "smtp.gmail.com"; properties.put("mail.smtp.starttls.enable", "true"); properties.put("mail.smtp.ssl.trust", host); properties.put("mail.smtp.user", from); properties.put("mail.smtp.password", pass); properties.put("mail.smtp.port", "587"); properties.put("mail.smtp.auth", "true"); Session session = Session.getDefaultInstance(properties); MimeMessage message = new MimeMessage(session); try { message.setFrom(new InternetAddress(from)); InternetAddress[] internetAddress = new InternetAddress[toAddress.length]; // iterate array of addresses for (int i = 0; i < toAddress.length; i++) { internetAddress[i] = new InternetAddress(toAddress[i]); } for (int i = 0; i < internetAddress.length; i++) { message.addRecipient(Message.RecipientType.TO, internetAddress[i]); } message.setSubject(subject); message.setText(body); Transport transport = session.getTransport("smtp"); transport.connect(host, from, pass); transport.sendMessage(message, message.getAllRecipients()); transport.close(); } catch (AddressException ae) { ae.printStackTrace(); } catch (MessagingException me) { me.printStackTrace(); } } }
b) Sending HTML email with JavaMail API
package com.javatips; import java.util.*; import javax.mail.*; import javax.mail.internet.*; public class SendHTMLEmail { public static void main(String[] args) { String from = "from@gmail.com"; String pass = "password"; String[] toAddress = new String[]{"to@gmail.com"}; String subject = "Java Mail Example"; String body = "<b>This is an example for HTML example</b>"; Properties properties = System.getProperties(); String host = "smtp.gmail.com"; properties.put("mail.smtp.starttls.enable", "true"); properties.put("mail.smtp.ssl.trust", host); properties.put("mail.smtp.user", from); properties.put("mail.smtp.password", pass); properties.put("mail.smtp.port", "587"); properties.put("mail.smtp.auth", "true"); Session session = Session.getDefaultInstance(properties); MimeMessage message = new MimeMessage(session); try { message.setFrom(new InternetAddress(from)); InternetAddress[] internetAddress = new InternetAddress[toAddress.length]; // iterate array of addresses for (int i = 0; i < toAddress.length; i++) { internetAddress[i] = new InternetAddress(toAddress[i]); } for (int i = 0; i < internetAddress.length; i++) { message.addRecipient(Message.RecipientType.TO, internetAddress[i]); } message.setSubject(subject); message.setContent(body, "text/html"); Transport transport = session.getTransport("smtp"); transport.connect(host, from, pass); transport.sendMessage(message, message.getAllRecipients()); transport.close(); } catch (AddressException ae) { ae.printStackTrace(); } catch (MessagingException me) { me.printStackTrace(); } } }
c) Sending email attachment with JavaMail API
package com.javatips; import java.util.*; import javax.activation.DataHandler; import javax.activation.DataSource; import javax.activation.FileDataSource; import javax.mail.*; import javax.mail.internet.*; public class SendAttachmentEmail { public static void main(String[] args) { String from = "from@gmail.com"; String pass = "password"; String[] toAddress = new String[] { "to@gmail.com" }; String subject = "Java Mail Example"; String body = "Hi, how are you"; Properties properties = System.getProperties(); String host = "smtp.gmail.com"; properties.put("mail.smtp.starttls.enable", "true"); properties.put("mail.smtp.ssl.trust", host); properties.put("mail.smtp.user", from); properties.put("mail.smtp.password", pass); properties.put("mail.smtp.port", "587"); properties.put("mail.smtp.auth", "true"); Session session = Session.getDefaultInstance(properties); MimeMessage message = new MimeMessage(session); try { message.setFrom(new InternetAddress(from)); InternetAddress[] internetAddress = new InternetAddress[toAddress.length]; // iterate array of addresses for (int i = 0; i < toAddress.length; i++) { internetAddress[i] = new InternetAddress(toAddress[i]); } for (int i = 0; i < internetAddress.length; i++) { message.addRecipient(Message.RecipientType.TO, internetAddress[i]); } message.setSubject(subject); message.setText(body); MimeBodyPart messageBodyPart = new MimeBodyPart(); Multipart multipart = new MimeMultipart(); String file = "exact path of file to be attached"; String fileName = "attachmentName"; DataSource source = new FileDataSource(file); messageBodyPart.setDataHandler(new DataHandler(source)); messageBodyPart.setFileName(fileName); multipart.addBodyPart(messageBodyPart); message.setContent(multipart); Transport transport = session.getTransport("smtp"); transport.connect(host, from, pass); transport.sendMessage(message, message.getAllRecipients()); transport.close(); } catch (AddressException ae) { ae.printStackTrace(); } catch (MessagingException me) { me.printStackTrace(); } } }