Convert Java Object To / From JSON, GSON Example
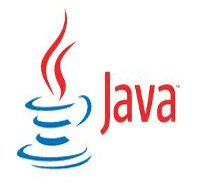
On this Simple GSON Example explains about converting a Java Object to JSON string nad JSON string to Java Object Using Google Gson JSON library
Google Gson is a Java library that can be used to convert Java Objects into their JSON representation. It can also be used to convert a JSON string to an equivalent Java object. Gson can work with arbitrary Java objects including pre-existing objects that you do not have source-code of.
Reference -> code.google.com/p/google-gson/
Here I am showing an example about How to convert a Java Object into JSON string and vice versa using Google Gson.
Required Libraries
Project structure
Student.java
Here I am creating a pojo class, this will be the converted in to JSON string.
public class Student {
private String firstName="Rockey";
private String lastName="Desousa";
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
@Override
public String toString() {
return "Student [firstName=" + firstName + ", lastName=" + lastName + "]";
}
}
Google Gson Example (Convert Java Object into JSON string)
For converting Java Student Object to JSON string, you can use following method
String jsonString = gson.toJson(student);
For converting JSON string to Java Student Object, you can use following method
student = gson.fromJson(jsonString, Student.class);
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
public class Main {
public static void main(String[] args) {
// Configure gson
GsonBuilder gsonBuilder = new GsonBuilder();
Gson gson = gsonBuilder.create();
Student student = new Student();
String jsonString = gson.toJson(student);
System.out.println(jsonString);
student = gson.fromJson(jsonString, Student.class);
System.out.println("student details" + student);
}
}
Output
{"firstName":"Rockey","lastName":"Desousa"} student details Student [firstName=Rockey, lastName=Desousa]
Google Gson JSON Pretty Print
If you need to format the JSON string, you can use the following code
gson = gsonBuilder.setPrettyPrinting().create();
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
public class Main {
public static void main(String[] args) {
// Configure gson
GsonBuilder gsonBuilder = new GsonBuilder();
Gson gson = gsonBuilder.create();
//set PrettyPrint
gson = gsonBuilder.setPrettyPrinting().create();
Student student = new Student();
String jsonString = gson.toJson(student);
System.out.println(jsonString);
Output
{ "firstName" : "Rockey", "lastName" : "Desousa" }