Convert Java InputStream to String
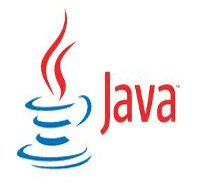
In this tutorial, I am showing how to convert Java InputStream to String using Plain Java, Java 8, Apache Commons IO library and Guava.
An InputStream
is connected to some data streams
like a file, memory
bufferes, network
connection, pipe etc. It is
used for reading the data from above sources.
You can also see an example for convert Java String to InputStream (java String to InputStream).
Convert InputStream to String Using JDK
import java.io.ByteArrayInputStream; import java.io.IOException; import java.io.InputStream; import java.nio.charset.StandardCharsets; import java.util.Scanner; public class Test { public static void main(String[] args) throws IOException { String hello = "hello world"; InputStream is = new ByteArrayInputStream( hello.getBytes(StandardCharsets.UTF_8) ); Scanner s = new Scanner(is).useDelimiter("\\A"); String result = s.hasNext() ? s.next() : ""; System.out.println(result); } }
In below example, we are using InputStreamReader for converting InputStream to string
import java.io.ByteArrayInputStream; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.io.Reader; import java.nio.charset.StandardCharsets; public class Test { public static void main(String[] args) throws IOException { String hello = "hello world"; InputStream is = new ByteArrayInputStream(hello.getBytes(StandardCharsets.UTF_8)); final int buffSize = 1024; final char[] buff = new char[buffSize]; final StringBuilder result = new StringBuilder(); Reader in = new InputStreamReader(is, "UTF-8"); int read; while ((read = in.read(buff, 0, buff.length)) != -1) { result.append(buff, 0, read); } System.out.println(result); } }
In below example, we are using ByteArrayOutputStream for converting InputStream to string
import java.io.ByteArrayInputStream; import java.io.ByteArrayOutputStream; import java.io.IOException; import java.io.InputStream; import java.nio.charset.StandardCharsets; public class Test { public static void main(String[] args) throws IOException { String hello = "hello world"; InputStream is = new ByteArrayInputStream(hello.getBytes(StandardCharsets.UTF_8)); ByteArrayOutputStream buffer = new ByteArrayOutputStream(); int read; byte[] buff = new byte[1024]; while ((read = is.read(buff, 0, buff.length)) != -1) { buffer.write(buff, 0, read); } buffer.flush(); byte[] byteArray = buffer.toByteArray(); String result = new String(byteArray, StandardCharsets.UTF_8); System.out.println(result); } }
Convert InputStream to String Using Java 8
import java.io.BufferedReader; import java.io.ByteArrayInputStream; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.nio.charset.StandardCharsets; import java.util.stream.Collectors; public class Test { public static void main(String[] args) throws IOException { String hello = "hello world"; InputStream is = new ByteArrayInputStream(hello.getBytes(StandardCharsets.UTF_8)); String result = new BufferedReader(new InputStreamReader(is)).lines().collect(Collectors.joining("\n")); System.out.println(result); } }
Convert InputStream to String Using Apache Commons IOUtils
import java.io.ByteArrayInputStream; import java.io.IOException; import java.io.InputStream; import java.nio.charset.StandardCharsets; import org.apache.commons.io.IOUtils; public class Test { public static void main(String[] args) throws IOException { String hello = "hello world"; InputStream is = new ByteArrayInputStream(hello.getBytes(StandardCharsets.UTF_8)); String result = IOUtils.toString(is, StandardCharsets.UTF_8); System.out.println(result); } }
In below example, you can also use IOUtils.copy of IOUtils to convert InputStream to String.
import java.io.ByteArrayInputStream; import java.io.IOException; import java.io.InputStream; import java.io.StringWriter; import java.nio.charset.StandardCharsets; import org.apache.commons.io.IOUtils; public class Test { public static void main(String[] args) throws IOException { String hello = "hello world"; InputStream is = new ByteArrayInputStream(hello.getBytes(StandardCharsets.UTF_8)); StringWriter result = new StringWriter(); IOUtils.copy(is, result, "UTF-8"); System.out.println(result); } }
Convert InputStream to String Using Guava
import java.io.ByteArrayInputStream; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.nio.charset.StandardCharsets; import com.google.common.base.Charsets; import com.google.common.io.CharStreams; public class Test { public static void main(String[] args) throws IOException { String hello = "hello world"; InputStream is = new ByteArrayInputStream(hello.getBytes(StandardCharsets.UTF_8)); String result = CharStreams.toString(new InputStreamReader( is, Charsets.UTF_8)); System.out.println(result); } }
Output
hello world