Jersey Bean Validation Example
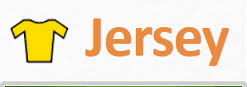
Jersey Bean Validation Example explains about Jersey bean valiation both input validations and service layer validations
JAX-RS is Java API for RESTful Webservices which is very rely upon Representational State Transfer model, you can view JAX-RS specification
JAX-RS uses annotations for simplifying the development efforts.
Now a days more & more deployment is going based on restful services compare to WSDL Webservices, due to the weights towards the simplicity of configuration
Bean Validation is a process of verifying that some data obeys one or more pre-defined constraints. The Bean Validation specification supports the use of constraint annotations as a way of declaratively validating beans, method parameters and method returned values. Below post describes support for Bean Validation in Jersey in terms of the needed dependencies, configuration, registration and usage
Reference -> https://jersey.java.net/documentation/latest/bean-validation.html
You can see the below example, which is demonstrating How to do bean validations using jersey 2
Required Libraries
You need to download
Following jar must be in classpath
- classmate-0.8.0.jar
- hibernate-validator-5.1.2.Final.jar
- hk2-api-2.4.0-b06.jar
- hk2-locator-2.4.0-b06.jar
- hk2-utils-2.4.0-b06.jar
- jackson-annotations-2.4.6.jar
- jackson-core-2.4.6.jar
- jackson-databind-2.4.6.jar
- jackson-jaxrs-base-2.3.2.jar
- jackson-jaxrs-json-provider-2.3.2.jar
- jackson-module-jaxb-annotations-2.3.2.jar
- javassist-3.18.1-GA.jar
- javax.annotation-api-1.2.jar
- javax.inject-2.4.0-b25.jar
- javax.ws.rs-api-2.0.1.jar
- jboss-logging-3.1.1.GA.jar
- jersey-bean-validation-2.19.jar
- jersey-client.jar
- jersey-common.jar
- jersey-container-servlet-core.jar
- jersey-container-servlet.jar
- jersey-guava-2.19.jar
- jersey-media-json-jackson-2.14.jar
- jersey-server.jar
- validation-api-1.1.0.Final.jar
Jersey Bean Validation Example
I am creating a sample restful service project that pass Student id and return the name of student. Here Jersey bean validation is using to check whether the student id is blank and also return if student is not exist. The service is using simple POJO (Plain Old Java Object) bean.
Firstly create a Dynamic Web Project (File->New->Dynamic Web Project) named "Jersey2Validation" according to following screenshot
Create a StudentID validator
Here StudentID interface is used for defining the logic for this service, inside isValid() method we are checking the conditions
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import javax.validation.Constraint;
import javax.validation.ConstraintValidator;
import javax.validation.ConstraintValidatorContext;
import javax.validation.Payload;
@Retention(RetentionPolicy.RUNTIME)
@Constraint(validatedBy = StudentID.StudentIDValidator.class)
public @interface StudentID {
String message() default "StudentID does not exist";
Class<?>[] groups() default {};
Class<? extends Payload>[] payload() default {};
public class StudentIDValidator implements ConstraintValidator<StudentID, String> {
@Override
public void initialize(final StudentID studentID) {
}
@Override
public boolean isValid(final String studentID, ConstraintValidatorContext ctx) {
return studentID != null;
}
}
}
Create a Controller
Inside StudentController, we are validating the student id and it should not be blank. if student id is blank it will return the message using Hibernate validator. Service also returns the error message if student is not exist corresponding to the student id
Here we are using one example showing with GET method & another with POST method
GET---> Calling this method will not result any changes to the server
POST---> Calling this method will result changes to the server, This have more secure than GET method
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
import org.hibernate.validator.constraints.NotBlank;
import com.student.validator.StudentID;
@Path("Services")
public class StudentController {
@POST
@Path("/changeName")
@StudentID
@Produces(MediaType.APPLICATION_JSON)
public String getStudentID(@NotBlank(message = "Search string cannot be empty") final String id) {
return findStudentByID(id);
}
// mocking the data, you need to retrieve from database
private String findStudentByID(String id) {
if ("1".equals(id)) {
return "Rockey";
} else {
return null;
}
}
}
@Consumes annotation specifies, the request is coming from the client
you can specify the Mime type as @Consumes("application/xml"), if the request is in xml format
@Produces annotation specifies, the response is going to the client
you can specify the Mime type as @Produces ("application/xml"), if the response need to be in xml format
Create ApplicationConfig
Here we register the StudentController class, so that jersey will invoke properly
import org.glassfish.jersey.server.ResourceConfig;
import com.controller.StudentController;
public class ApplicationConfig extends ResourceConfig {
public ApplicationConfig() {
register(StudentController.class);
}
}
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1"> <display-name>Jersey2Validation</display-name> <servlet> <servlet-name>jersey</servlet-name> <servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class> <init-param> <param-name>javax.ws.rs.Application</param-name> <param-value>com.config.ApplicationConfig</param-value> </init-param> <init-param> <param-name>jersey.config.beanValidation.enableOutputValidationErrorEntity.server</param-name> <param-value>true</param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>jersey</servlet-name> <url-pattern>/rest/*</url-pattern> </servlet-mapping> </web-app>
Publishing Jersey 2 Restful Service
Jersey Bean Validation Output When Student ID Blank
Jersey Bean Validation Output When Valid Student ID
Jersey Bean Validation Output When Student Not Exists
Here there is no student exists corresponding to studentid 2