Iterate HashMap Using Java
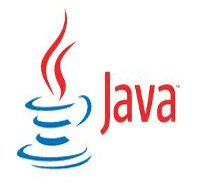
In this tutorial, I am showing how to iterate through a HashMap using java in different useful ways.
If you are using Java 8 for the map iteration, you can use lambda expression as like method 5.
You can also use with generics as method 3, and without generics as method 4, if you are using old versions of jdk.
1) Iterate entries using ForEach loop
import java.io.IOException; import java.util.HashMap; import java.util.Map; public class Test { public static void main(String[] args) throws IOException { Map<Integer, Integer> map = new HashMap<Integer, Integer>(); map.put(1, 10); map.put(2, 20); // Iterating entries using a For Each loop for (Map.Entry<Integer, Integer> entry : map.entrySet()) { System.out.println("Key = " + entry.getKey() + ", Value = " + entry.getValue()); } } }
2) Iterate keys / values using ForEach loop
If you only need to iterate either keys or values only, you can use map.keySet() and map.values() import java.io.IOException; import java.util.HashMap; import java.util.Map; public class Test { public static void main(String[] args) throws IOException { Map<Integer, Integer> map = new HashMap<Integer, Integer>(); map.put(1, 10); map.put(2, 20); // Iterating over keys for (Integer key : map.keySet()) { System.out.println("Key = " + key); } // Iterating over values for (Integer value : map.values()) { System.out.println("Value = " + value); } } }
3) Iterate using Iterator with generics
import java.io.IOException; import java.util.HashMap; import java.util.Iterator; import java.util.Map; public class Test { public static void main(String[] args) throws IOException { Map<Integer, Integer> map = new HashMap<Integer, Integer>(); map.put(1, 10); map.put(2, 20); Iterator<Map.Entry<Integer, Integer>> entries = map.entrySet().iterator(); while (entries.hasNext()) { Map.Entry<Integer, Integer> entry = entries.next(); System.out.println("Key = " + entry.getKey() + ", Value = " + entry.getValue()); } } }
4) Iterate using Iterator without generics
import java.io.IOException; import java.util.HashMap; import java.util.Iterator; import java.util.Map; public class Test { public static void main(String[] args) throws IOException { Map map = new HashMap(); map.put(1, 10); map.put(2, 20); Iterator<Map.Entry> entries = map.entrySet().iterator(); while (entries.hasNext()) { Map.Entry entry = (Map.Entry) entries.next(); Integer key = (Integer) entry.getKey(); Integer value = (Integer) entry.getValue(); System.out.println("Key = " + key + ", Value = " + value); } } }
5) Iterate using Java 8 Lambda Expression
import java.io.IOException; import java.util.HashMap; import java.util.Map; public class Test { public static void main(String[] args) throws IOException { Map<Integer, Integer> map = new HashMap<Integer, Integer>(); map.put(1, 10); map.put(2, 20); map.forEach((k, v) -> System.out.println("key: " + k + " value:" + v)); } }Output
key: 1 value:10 key: 2 value:20