Create ArrayList from Array In Java
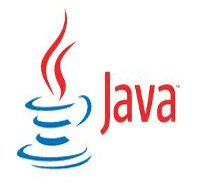
In this tutorial, I am showing how to Create ArrayList from array using java in different useful ways.
1) You can use Arrays.asList(array) to convert an array to an
Arraylist.
2) If you are working with java 8 you can use stream
api Stream.of(array).collect(Collectors.toCollection(ArrayList::new))
3) If you are working with java 9 you can use List.of("Hello", "World"), where this list is backed by an array.
Create ArrayList from array
import java.io.IOException; import java.util.ArrayList; import java.util.Arrays; import java.util.stream.Collectors; import java.util.stream.Stream; public class Main { public static void main(String[] args) throws IOException { String[] array = { "Hello", "World" }; // Create ArrayList from array ArrayList<String> list = new ArrayList<>(Arrays.asList(array)); System.out.println(list); // In Java 8 you can use list = Stream.of(array).collect(Collectors.toCollection(ArrayList::new)); System.out.println(list); // In Java 9 you can use List<String> list = List.of("Hello", "World"); System.out.println(list); // Initialization of an ArrayList in one line ArrayList<String> list = new ArrayList<String>() { { add("Hello"); add("World"); } }; } }
output
[Hello, World]
1 Responses to "Create ArrayList from Array In Java"