Resolve CXF JSON Single Element Array Issue
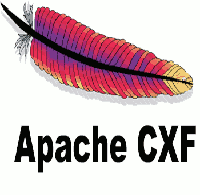
Resolve CXF JSON Single Element Array Issue explains about How to resolve the issue on array serialization when using jettison library
Jettison is an open source java library for processing JSON format. It is the default library bundled with CXF framework, the problem with jettison is that if an array / list having multiple items jettison serialize the array correctly, but it have single item it will serialize incorrectly. you can see the below example
length = 1: field = value length > 1: field = [value1, value2, ...]
you can see if it have one item enclosing bracket is missing. In order to avoid this issue, I am going to use Jackson library by replacing Jettison
I am going to reuse Apache CXF With Jackson just change the Student object in order to add a List with single element
Create a Student Object
import java.util.List;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement(name = "Student")
public class Student {
private String name;
private List addressList;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public List getAddressList() {
return addressList;
}
public void setAddressList(List addressList) {
this.addressList = addressList;
}
}
Modify ChangeStudentDetailsImpl
Here I am creating a single element ArrayList, just for showing the issue
import java.util.ArrayList;
import javax.ws.rs.Consumes;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
@Consumes("application/json")
@Produces("application/json")
public class ChangeStudentDetailsImpl implements ChangeStudentDetails {
@POST
@Path("/changeName")
public Student changeName(Student student) {
student.setName("HELLO " + student.getName());
ArrayList addressList = new ArrayList();
addressList.add("Bangalore");
student.setAddressList(addressList);
return student;
}
@GET
@Path("/getName")
public Student getName() {
Student student = new Student();
student.setName("Rockey");
ArrayList addressList = new ArrayList();
addressList.add("Pune");
return student;
}
}
you can also see CXF Restful Client in order to run this restful service
Output when invoking the POST method using jettison
{"Student":{"name":"HELLO Tom","addressList":"Bangalore"}}
Output when invoking the POST method using Jackson
{"Student":{"name":"HELLO Tom","addressList":["Bangalore"]}}
From the above output you can see that enclosing bracket is missing on jettison library while it is available on Jackson library