Java Persistence (JPA) Tutorial With EclipseLink
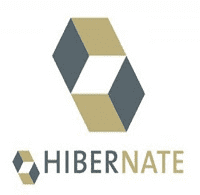
JPA Tutorial With EclipseLink explains step by step details of setting / configuring Java Persistence (JPA) With EclipseLink And Eclipse
How To Configure EclipseLink (JPA) With Oracle Database?
Java Persistence API (JPA), is a standard interface which wraps different ORM tools such as Hibernate, EclipseLink, OpenJPA etc. The benefits of using JPA is that you can change the underlying implementation of without changing any code.
ie; you can able to change Hibernate implementation to EclipseLink implementation without changing the code base.
On this standalone JPA Example, we are using Java Persistence (JPA) With EclipseLink
EclipseLink is popular open source ORM (Object Relation Mapping) tool for Java platform, for mapping an entity to a traditional relational database like Oracle, MySQL etc
By using JPA, we can do database operations like Create, Read, Update & Delete etc, with very little code.
Required Libraries
You need to download
Following jar must be in classpath
- eclipselink-jpa-modelgen_2.4.1.v20121003-ad44345.jar
- classes12.jar
- javax.persistence_1.0.0.jar
- javax.persistence_2.0.4.v201112161009.jar
- org.eclipse.persistence.jpars_2.4.1.v20121003-ad44345.jar
- org.eclipse.persistence.jpars.source_2.4.1.v20121003-ad44345.jar
I am creating a sample project that persists Student object (simple POJO (Plain Old Java Object)) into database using JPA
Firstly create a Java Project (File->New->Project), select Java Project and click next, provide name as "JPA2Example" according to following screenshot
persistence.xml
persistence.xml file must be under src/META-INF (Please check below screenshot(project structure)
<persistence xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/persistence http://java.sun.com/xml/ns/persistence/persistence_2_0.xsd" version="2.0" xmlns="http://java.sun.com/xml/ns/persistence"> <persistence-unit name="test" transaction-type="RESOURCE_LOCAL"> <class>com.test.jpa.Student</class> <properties> <property name="javax.persistence.jdbc.driver" value="oracle.jdbc.driver.OracleDriver" /> <property name="javax.persistence.jdbc.url" value="jdbc:oracle:thin:@192.148.27.140:1521:database" /> <property name="javax.persistence.jdbc.user" value="username" /> <property name="javax.persistence.jdbc.password" value="password" /> <property name="eclipselink.ddl-generation" value="create-tables" /> <property name="eclipselink.ddl-generation.output-mode" value="database" /> </properties> </persistence-unit> </persistence>
Create a Student Object(Student.java)
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.Table;
@Entity
@Table(name = "STUDENT")
public class Student implements java.io.Serializable {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
@Column(name = "STUDENTID")
private long studentId;
@Column(name = "STUDENTNAME")
private String studentName;
public void setStudentId(long studentId) {
this.studentId = studentId;
}
public long getStudentId() {
return studentId;
}
public void setStudentName(String studentName) {
this.studentName = studentName;
}
public String getStudentName() {
return studentName;
}
}
@Entity declares the class as an entity (i.e. a persistent POJO class)
@Table is set at the class level; it allows you to define the table, catalog, and schema names for your entity mapping. If no @Table is defined the default values are used: the unqualified class name of the entity.
@Id declares the identifier property of this entity.
@GeneratedValue annotation is used to specify the primary key generation strategy to use. If the strategy is not specified by default AUTO will be used.
@Column annotation is used to specify the details of the column to which a field or property will be mapped. If the @Column annotation is not specified by default the property name will be used as the column name.
EntityManagerUtil.java
import javax.persistence.EntityManager;
import javax.persistence.EntityManagerFactory;
import javax.persistence.Persistence;
public class EntityManagerUtil {
private static final EntityManagerFactory entityManagerFactory;
static {
try {
entityManagerFactory = Persistence.createEntityManagerFactory("test");
} catch (Throwable ex) {
System.err.println("Initial SessionFactory creation failed." + ex);
throw new ExceptionInInitializerError(ex);
}
}
public static EntityManager getEntityManager() {
return entityManagerFactory.createEntityManager();
}
}
Package Structure
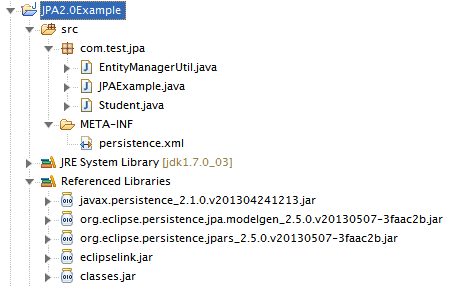
Testing (JPAExample.java)
import java.util.Iterator;
import java.util.List;
import javax.persistence.EntityManager;
public class JPAExample {
private EntityManager entityManager = EntityManagerUtil.getEntityManager();
public static void main(String[] args) {
JPAExample example = new JPAExample();
System.out.println("After Sucessfully insertion ");
Student student1 = example.saveStudent("Sumith");
Student student2 = example.saveStudent("Anoop");
example.listStudent();
System.out.println("After Sucessfully modification ");
example.updateStudent(student1.getStudentId(), "Sumith Honai");
example.updateStudent(student2.getStudentId(), "Anoop Pavanai");
example.listStudent();
System.out.println("After Sucessfully deletion ");
example.deleteStudent(student2.getStudentId());
example.listStudent();
}
public Student saveStudent(String studentName) {
Student student = new Student();
try {
entityManager.getTransaction().begin();
student.setStudentName(studentName);
student = entityManager.merge(student);
entityManager.getTransaction().commit();
} catch (Exception e) {
entityManager.getTransaction().rollback();
}
return student;
}
public void listStudent() {
try {
entityManager.getTransaction().begin();
@SuppressWarnings("unchecked")
List<Student> Students = entityManager.createQuery("from Student").getResultList();
for (Iterator<Student> iterator = Students.iterator(); iterator.hasNext();) {
Student student = (Student) iterator.next();
System.out.println(student.getStudentName());
}
entityManager.getTransaction().commit();
} catch (Exception e) {
entityManager.getTransaction().rollback();
}
}
public void updateStudent(Long studentId, String studentName) {
try {
entityManager.getTransaction().begin();
Student student = (Student) entityManager.find(Student.class, studentId);
student.setStudentName(studentName);
entityManager.getTransaction().commit();
} catch (Exception e) {
entityManager.getTransaction().rollback();
}
}
public void deleteStudent(Long studentId) {
try {
entityManager.getTransaction().begin();
Student student = (Student) entityManager.find(Student.class, studentId);
entityManager.remove(student);
entityManager.getTransaction().commit();
} catch (Exception e) {
entityManager.getTransaction().rollback();
}
}
}
Output
After Sucessfully insertion Sumith Anoop After Sucessfully modification Sumith Honai Anoop Pavanai After Sucessfully deletion Sumith Honai