EclipseLink JPA With SQL Server
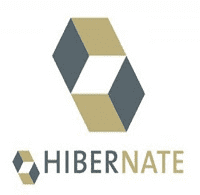
EclipseLink JPA With SQL Server explains step by step details of setting / configuring Java Persistence JPA With EclipseLink And SQL Server
How To Configure EclipseLink JPA With SQL Server?
Java Persistence API, is a standard interface which wraps different ORM tools such as Hibernate, EclipseLink, OpenJPA etc.
ie; you can able to change Hibernate implementation to EclipseLink implementation without changing the code base.
On this standalone JPA Example, we are using EclipseLink With SQL Server
If you want to configure EclipseLink JPA With Oracle, you can follow JPA Tutorial With EclipseLink
EclipseLink is popular open source ORM (Object Relation Mapping) tool for Java platform, for mapping an entity to a traditional relational like Oracle, MySQL, SQL Server etc
I am going to re-use JPA Tutorial With EclipseLink
You can check below different database with Hibernate and EclipseLink examples
Modify persistence.xml
persistence.xml file must be under src/META-INF (Please check below screenshot(project structure)
<persistence xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/persistence http://java.sun.com/xml/ns/persistence/persistence_2_0.xsd" version="2.0" xmlns="http://java.sun.com/xml/ns/persistence"> <persistence-unit name="test" transaction-type="RESOURCE_LOCAL"> <class>com.test.jpa.Student</class> <properties> <property name="javax.persistence.jdbc.driver" value="com.microsoft.sqlserver.jdbc.SQLServerDriver" /> <property name="javax.persistence.jdbc.url" value="jdbc:sqlserver://[serverName[\instanceName][:portNumber]];databaseName=<databaseName>" /> <property name="javax.persistence.jdbc.user" value="username" /> <property name="javax.persistence.jdbc.password" value="password" /> <property name="eclipselink.ddl-generation" value="create-tables" /> <property name="eclipselink.ddl-generation.output-mode" value="database" /> </properties> </persistence-unit> </persistence>
Package Structure
Now package structure looks like following
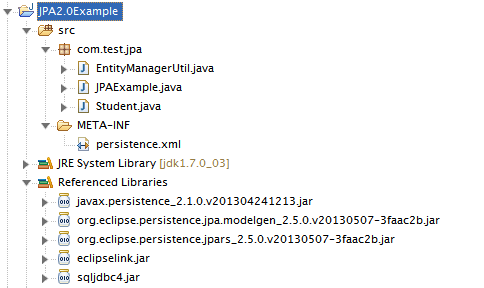
Testing (JPAExample.java)
import java.util.Iterator;
import java.util.List;
import javax.persistence.EntityManager;
//EclipseLink JPA With SQL Server Example
public class JPAExample {
private EntityManager entityManager = EntityManagerUtil.getEntityManager();
public static void main(String[] args) {
JPAExample example = new JPAExample();
System.out.println("After Sucessfully insertion ");
Student student1 = example.saveStudent("Sumith");
Student student2 = example.saveStudent("Anoop");
example.listStudent();
System.out.println("After Sucessfully modification ");
example.updateStudent(student1.getStudentId(), "Sumith Honai");
example.updateStudent(student2.getStudentId(), "Anoop Pavanai");
example.listStudent();
System.out.println("After Sucessfully deletion ");
example.deleteStudent(student2.getStudentId());
example.listStudent();
}
public Student saveStudent(String studentName) {
Student student = new Student();
try {
entityManager.getTransaction().begin();
student.setStudentName(studentName);
student = entityManager.merge(student);
entityManager.getTransaction().commit();
} catch (Exception e) {
entityManager.getTransaction().rollback();
}
return student;
}
public void listStudent() {
try {
entityManager.getTransaction().begin();
@SuppressWarnings("unchecked")
List<Student> Students = entityManager.createQuery("from Student").getResultList();
for (Iterator<Student> iterator = Students.iterator(); iterator.hasNext();) {
Student student = (Student) iterator.next();
System.out.println(student.getStudentName());
}
entityManager.getTransaction().commit();
} catch (Exception e) {
entityManager.getTransaction().rollback();
}
}
public void updateStudent(Long studentId, String studentName) {
try {
entityManager.getTransaction().begin();
Student student = (Student) entityManager.find(Student.class, studentId);
student.setStudentName(studentName);
entityManager.getTransaction().commit();
} catch (Exception e) {
entityManager.getTransaction().rollback();
}
}
public void deleteStudent(Long studentId) {
try {
entityManager.getTransaction().begin();
Student student = (Student) entityManager.find(Student.class, studentId);
entityManager.remove(student);
entityManager.getTransaction().commit();
} catch (Exception e) {
entityManager.getTransaction().rollback();
}
}
}
Output
After Sucessfully insertion Sumith Anoop After Sucessfully modification Sumith Honai Anoop Pavanai After Sucessfully deletion Sumith Honai