Hibernate JPA One To Many Relation Mapping Example
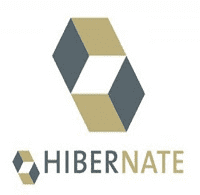
Hibernate JPA One To Many Relation Mapping Example explains about how to implement a One To Many relationship by using Hibernate JPA
Java Persistence API (JPA) is a standard interface which wraps different ORM tools such as Hibernate, EclipseLink, OpenJPA etc. ie; you can able to change Hibernate implementation to EclipseLink implementation without changing the code base.
one-to-many entity relationship, each record of an entity have multiple records in other associated entity
On this standalone One To Many Mapping Example, we are using Java Persistence (JPA) With MySQL Database. If you want to configure Hibernate JPA With other Databases, you can check below table
Let us see how to implement JPA One-to-Many relationship using Hibernate
Required Libraries
You need to download
Following jar must be in classpath
- antlr-2.7.7.jar
- dom4j-1.6.1.jar
- hibernate-commons-annotations-4.0.4.Final.jar
- hibernate-core-4.3.1.Final.jar
- hibernate-entitymanager-4.3.1.Final.jar
- hibernate-jpa-2.1-api-1.0.0.Final.jar
- jandex-1.1.0.Final.jar
- dom4j-1.6.1.jar
- jboss-logging-3.1.3.GA.jar
- jboss-transaction-api_1.2_spec-1.0.0.Final.jar
- mysql-connector-java-5.1.28-bin.jar
Table structure
CREATE TABLE `company` ( `ADDRESSID` bigint(20) NOT NULL AUTO_INCREMENT, `CITY` varchar(255) DEFAULT NULL, `COUNTRY` varchar(255) DEFAULT NULL, `STATE` varchar(255) DEFAULT NULL, `STREET` varchar(255) DEFAULT NULL, PRIMARY KEY (`ADDRESSID`) ); CREATE TABLE `employee` ( `EMPLOYEEID` bigint(20) NOT NULL AUTO_INCREMENT, `EMPLOYEENAME` varchar(255) DEFAULT NULL, `COMPANY_EMPLOYEE` bigint(20) DEFAULT NULL, PRIMARY KEY (`EMPLOYEEID`), KEY `FK_j1a2fo8yfvds4h8fr03ydga18` (`COMPANY_EMPLOYEE`), CONSTRAINT `FK_j1a2fo8yfvds4h8fr03ydga18` FOREIGN KEY (`COMPANY_EMPLOYEE`) REFERENCES `company` (`ADDRESSID`) ) ;
Create Company & Employee model classes as per the above table structure
Company.java
import java.util.Set;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.OneToMany;
@Entity
public class Company implements java.io.Serializable {
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue
@Column(name = "ADDRESSID")
private long addressID;
@Column(name = "STREET")
private String street;
@Column(name = "CITY")
private String city;
@Column(name = "STATE")
private String state;
@Column(name = "COUNTRY")
private String country;
@OneToMany(cascade = CascadeType.ALL,fetch=FetchType.LAZY)
@JoinColumn(name = "COMPANY_EMPLOYEE")
private Set<Employee> employees;
public Company() {
super();
}
public Company(String street, String city, String state, String country) {
this.street = street;
this.city = city;
this.state = state;
this.country = country;
}
public long getAddressID() {
return addressID;
}
public void setAddressID(long addressID) {
this.addressID = addressID;
}
public String getStreet() {
return street;
}
public void setStreet(String street) {
this.street = street;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
public Set<Employee> getEmployees() {
return employees;
}
public void setEmployees(Set<Employee> employees) {
this.employees = employees;
}
}
Employee.java
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
@Entity
public class Employee implements java.io.Serializable {
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue
@Column(name = "EMPLOYEEID")
private long employeeId;
@Column(name = "EMPLOYEENAME")
private String employeeName;
public Employee() {
super();
}
public Employee(String employeeName) {
super();
this.employeeName = employeeName;
}
public Employee(String employeeName, Company company) {
this.employeeName = employeeName;
}
public long getEmployeeId() {
return employeeId;
}
public void setEmployeeId(long employeeId) {
this.employeeId = employeeId;
}
public String getEmployeeName() {
return employeeName;
}
public void setEmployeeName(String employeeName) {
this.employeeName = employeeName;
}
}
@OneToMany(cascade = CascadeType.ALL,fetch=FetchType.LAZY) annotation is used for linking single record of Employee table with multiple records of Company table
@JoinColumn(name = "COMPANY_EMPLOYEE") , which is connecting company & employee tables by using keyword @JoinColumn (COMPANY_EMPLOYEE)
@Entity declares the class as an entity (i.e. a persistent POJO class)
@Table is set at the class level; it allows you to define the table, catalog, and schema names for your entity mapping. If no @Table is defined the default values are used: the unqualified class name of the entity.
@Id declares the identifier property of this entity.
@GeneratedValue annotation is used to specify the primary key generation strategy to use. If the strategy is not specified by default AUTO will be used.
@Column annotation is used to specify the details of the column to which a field or property will be mapped. If the @Column annotation is not specified by default the property name will be used as the column name.
EntityManagerUtil.java
import javax.persistence.EntityManager;
import javax.persistence.EntityManagerFactory;
import javax.persistence.Persistence;
public class EntityManagerUtil {
private static final EntityManagerFactory entityManagerFactory;
static {
try {
entityManagerFactory = Persistence.createEntityManagerFactory("test");
} catch (Throwable ex) {
System.err.println("Initial SessionFactory creation failed." + ex);
throw new ExceptionInInitializerError(ex);
}
}
public static EntityManager getEntityManager() {
return entityManagerFactory.createEntityManager();
}
}
persistence.xml
persistence.xml file must be under src/META-INF Please check the screenshot(project structure)
<persistence xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/persistence http://java.sun.com/xml/ns/persistence/persistence_2_0.xsd" version="2.0" xmlns="http://java.sun.com/xml/ns/persistence"> <persistence-unit name="test" transaction-type="RESOURCE_LOCAL"> <properties> <property name="javax.persistence.jdbc.driver" value="com.mysql.jdbc.Driver" /> <property name="javax.persistence.jdbc.url" value="jdbc:mysql://localhost:3306/test" /> <property name="javax.persistence.jdbc.user" value="root" /> <property name="javax.persistence.jdbc.password" value="root" /> <property name="hibernate.hbm2ddl.auto" value="create-drop" /> <property name="hibernate.dialect" value="org.hibernate.dialect.MySQLDialect" /> </properties> </persistence-unit> </persistence>
Project Structure
Now project structure looks like following
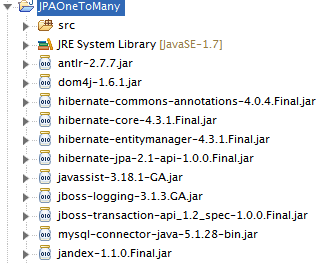
Just execute below class and see the output
JPAOneToManyExample.java
import java.util.HashSet;
import java.util.Set;
import javax.persistence.EntityManager;
public class JPAOneToManyExample {
public static void main(String[] args) {
EntityManager entityManager = EntityManagerUtil.getEntityManager();
try {
entityManager.getTransaction().begin();
Company company = new Company();
company.setCity("Kolkata");
company.setCountry("India");
company.setState("Bengal");
company.setStreet("Number 15");
Employee employee1 = new Employee("Rockey");
Employee employee2 = new Employee("Jose");
Set<Employee> employees = new HashSet<Employee>();
employees.add(employee1);
employees.add(employee2);
company.setEmployees(employees);
entityManager.persist(company);
entityManager.getTransaction().commit();
} catch (Exception e) {
entityManager.getTransaction().rollback();
}finally {
entityManager.close();
}
}
}
Output
ADDRESSID | CITY | COUNTRY | STATE | STREET |
1 | Kolkata | India | Bengal | Number 15 |
EMPLOYEEID | EMPLOYEENAME | COMPANY_EMPLOYEE |
1 | Rockey | 1 |
2 | Jose | 1 |