How To Maintain Cookies Using CXF
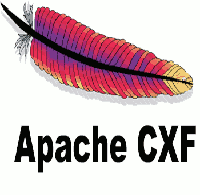
How To Maintain Cookies Using CXF explains about Maintaining Cookies Using CXF
I am showing here, How to do maintain Cookies in CXF framework, this is applicable for both SOAP & RESTFul services
Message message = PhaseInterceptorChain.getCurrentMessage(); HttpServletRequest request = (HttpServletRequest)message.get(AbstractHTTPDestination.HTTP_REQUEST); Cookie[] cookies = request.getCookies();
On this code, we are getting HttpServletRequest and from that we are getting cookies object in CXF
You can see the below example, on which we are storing a value into cookies from client side and retrieving the same, using CXF Framework
Enable Cookies Using CXF
I am going to re-use CXF Restful Tutorial
We are making changes ChangeStudentDetailsImpl class, just adding
PhaseInterceptorChain.getCurrentMessage() and getting HttpServletRequest, so that we can access Cookie object in CXF Framework
package com.student;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServletRequest;
import javax.ws.rs.Consumes;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import org.apache.cxf.message.Message;
import org.apache.cxf.phase.PhaseInterceptorChain;
import org.apache.cxf.transport.http.AbstractHTTPDestination;
// Maintain Cookies Using CXF
@Consumes("application/json")
@Produces("application/json")
public class ChangeStudentDetailsImpl implements ChangeStudentDetails {
@POST
@Path("/changeName")
public Student changeName(Student student) {
// Here We are getting cookies from HttpServletRequest
Message message = PhaseInterceptorChain.getCurrentMessage();
HttpServletRequest request = (HttpServletRequest) message.get(AbstractHTTPDestination.HTTP_REQUEST);
Cookie[] cookies = request.getCookies();
if (cookies != null) {
for (Cookie cookie : cookies) {
student.setName("HELLO " + cookie.getValue());
}
}
return student;
}
@GET
@Path("/getName")
public Student getName() {
Student student = new Student();
student.setName("Rockey");
return student;
}
}
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServletRequest;
import javax.ws.rs.Consumes;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import org.apache.cxf.message.Message;
import org.apache.cxf.phase.PhaseInterceptorChain;
import org.apache.cxf.transport.http.AbstractHTTPDestination;
// Maintain Cookies Using CXF
@Consumes("application/json")
@Produces("application/json")
public class ChangeStudentDetailsImpl implements ChangeStudentDetails {
@POST
@Path("/changeName")
public Student changeName(Student student) {
// Here We are getting cookies from HttpServletRequest
Message message = PhaseInterceptorChain.getCurrentMessage();
HttpServletRequest request = (HttpServletRequest) message.get(AbstractHTTPDestination.HTTP_REQUEST);
Cookie[] cookies = request.getCookies();
if (cookies != null) {
for (Cookie cookie : cookies) {
student.setName("HELLO " + cookie.getValue());
}
}
return student;
}
@GET
@Path("/getName")
public Student getName() {
Student student = new Student();
student.setName("Rockey");
return student;
}
}
Run Client
package com.client;
import java.io.IOException;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Scanner;
// Cookie Handling Using CXF
public class PostStudentClient {
public static void main(String[] args) {
try {
URL url = new URL("http://localhost:8080/CXFRestfulTutorial/rest/changeName");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(true);
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/json");
conn.setRequestProperty("Cookie", "name=Tom");
String input = "{\"Student\":{\"name\":\"Tom\"}}";
OutputStream os = conn.getOutputStream();
os.write(input.getBytes());
os.flush();
Scanner scanner;
String response;
if (conn.getResponseCode() != 200) {
scanner = new Scanner(conn.getErrorStream());
response = "Error From Server \n\n";
} else {
scanner = new Scanner(conn.getInputStream());
response = "Response From Server \n\n";
}
scanner.useDelimiter("\\Z");
System.out.println(response + scanner.next());
scanner.close();
conn.disconnect();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
import java.io.IOException;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Scanner;
// Cookie Handling Using CXF
public class PostStudentClient {
public static void main(String[] args) {
try {
URL url = new URL("http://localhost:8080/CXFRestfulTutorial/rest/changeName");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(true);
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/json");
conn.setRequestProperty("Cookie", "name=Tom");
String input = "{\"Student\":{\"name\":\"Tom\"}}";
OutputStream os = conn.getOutputStream();
os.write(input.getBytes());
os.flush();
Scanner scanner;
String response;
if (conn.getResponseCode() != 200) {
scanner = new Scanner(conn.getErrorStream());
response = "Error From Server \n\n";
} else {
scanner = new Scanner(conn.getInputStream());
response = "Response From Server \n\n";
}
scanner.useDelimiter("\\Z");
System.out.println(response + scanner.next());
scanner.close();
conn.disconnect();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output
Response From Server {"Student":{"name":"HELLO Tom"}}