Convert String To UpperCase In Java
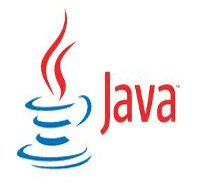
Convert String to UpperCase in Java Example describes about Converting a String to UpperCase in Java.
For converting a string to uppercase in java, you can use the following method of String class
String toUpperCase() will transforms characters of a particular String into upper case characters according to the current locale.
String toUpperCase(Locale locale)method will transforms all the characters of a particular String into upper case characters according to the given Locale specified.
Convert char To String in Java
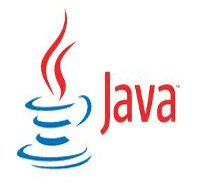
Convert char To String in Java Example describes about How To Convert char To String in Java.
Character class helps to wrap the primitive type value of char in an object. It also provides several utility functions for determining whether a character is lowercase, uppercase, digit, etc.
In addition, Character class provides several useful methods for dealing with char. When you need to Convert char To String in Java, we can use Character.toString(char c) method of Character class, It will convert the char to String.
If you need an Character object in lieu of primitive char, you can use Character.valueOf(char c). It will convert the char primitive to Character Object
Convert String to LowerCase in Java
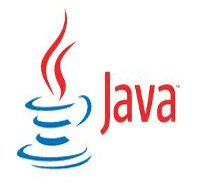
Convert String to LowerCase in Java Example describes about Converting a String to LowerCase in Java.
For converting a string to lowercase in java, you can use the following method of String class
String toLowerCase() will transforms all characters of a particular String into lower case characters according to the current locale.
String toLowerCase(Locale locale)method will transforms all characters of a particular String into lower case characters according to the given Locale specified.
Convert Java Primitive to String
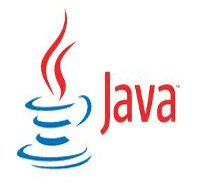
Convert Java Primitive to String Example describes about Converting a Primitive Type Value To String In Java.
There are two ways, you can able to convert java primitive type value into a string
- Use String.valueOf() method of String class
- Using string concatenation operator '+'.
Convert Char array to String in Java
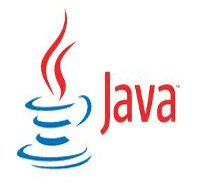
Convert Char array to String in Java Example describes about Convert Char array to String in Java.
String class have the below constructor
String string = new String(char[] value)
By using this constructor, you can easily build a string with provided characters
Convert byte to String in Java
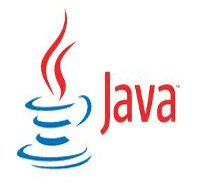
Convert byte to String in Java Example describes about Convert byte to String in Java.
Byte class helps to wrap the primitive type value of byte in an object
In addition, Byte class provides several useful methods for dealing with a byte primitives.
When you need to Convert byte to String in Java, we can use Byte.toString(byte b) method of Byte class, This will convert the byte to String.
If you need a Byte object in lieu of primitive byte, you can use method Byte.valueOf(byte b). This method will convert the byte primitive to Byte Object
Convert String to int in Java
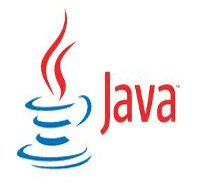
Convert String to int in Java Example describes about Converting a String to int using Java.
The Integer class will wraps the value of the primitive type int in an object. An Integer is an Object that contains a single int field. Integer values are immutable. If you want to change the value of a Integer variable, you need to create a new Integer object and discard the old one.
When you need to convert String to int in Java, we can use Integer.parseInt(String s) method of the Integer class, It will convert the string to primitive int.
If you need an Integer object in lieu of primitive int, you can use Integer.valueOf(String s). It will convert the string to Integer Object
In the following case If String is not Integer type, it will throws a NumberFormatException, so you should catch this exception
Convert long to String in Java
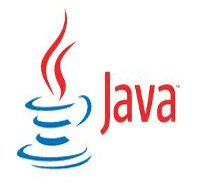
Convert long to String in Java Example describes about Convert long to String in Java.
Long class helps to wrap the primitive type value of long in an object.
In addition, Long class provides several useful methods for dealing with long primitives.
When we need to Convert long to String in Java, we can use Long.toString(long l) method of Long class, This method will convert the long to String.
If you need a Long type in lieu of primitive long, you can use the method Long.valueOf(long l). This method will convert the long primitive to Long type