Hibernate Query Tutorial
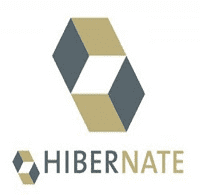
Hibernate Query Tutorial explains step by step details of setting / configuring Hibernate Query with Eclipse
Hibernate is popular open source ORM (Object Relation Mapping) tool for Java platform, for mapping an entity to a traditional relational database like Oracle, MySQL etc
By using Hibernate, we can done database operations like Create, Read, Update & Delete etc, with very little code.
On this Tutorial, You can see Read, Update & Delete operations by using Hibernate Query Language
You can also view some examples of a) Hibernate Tutorial b) Hibernate Annotations Tutorial
Required Libraries
You need to download
Following jar must be in classpath
- antlr-2.7.6.jar
- commons-collections-3.1.jar
- javassist-3.12.0.GA.jar
- jta-1.1.jar
- hibernate-jpa-2.0-api-1.0.1.Final.jar
- hibernate3.jar
- mysql-connector-java-5.1.18-bin.jar
- dom4j-1.6.1.jar
- slf4j-api-1.6.1.jar
I am creating a sample project that persists Student object (simple POJO (Plain Old Java Object)) into database using hibernate.
Firstly create a Java Project (File->New->Project), select Java Project and click next, provide name as "HibernateExample" according to following screenshot
hibernate.cfg.xml
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name="hibernate.connection.driver_class">com.mysql.jdbc.Driver</property> <property name="hibernate.connection.url">jdbc:mysql://localhost/test</property> <property name="hibernate.connection.username">root</property> <property name="connection.password">root</property> <property name="connection.pool_size">1</property> <property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property> <property name="show_sql">true</property> <property name="hbm2ddl.auto">create</property> <mapping class="com.javatips.student.Student"/> </session-factory> </hibernate-configuration>
HibernateUtil.java
HibernateUtil class will help you for creating the SessionFactory from the Hibernate configuration file. The implementation is shown bellow:
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class HibernateUtil {
private static final SessionFactory sessionFactory;
static {
try {
sessionFactory = new Configuration().configure().buildSessionFactory();
} catch (Throwable ex) {
System.err.println("Initial SessionFactory creation failed." + ex);
throw new ExceptionInInitializerError(ex);
}
}
public static SessionFactory getSessionFactory() {
return sessionFactory;
}
}
Create a Student Object(Student.java)
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.Table;
@Entity
@Table(name="StudentDetails")
public class Student implements java.io.Serializable {
private long studentId;
private String studentName;
public void setStudentId(long studentId) {
this.studentId = studentId;
}
@Id
@GeneratedValue
@Column(name="STUDENT_ID")
public long getStudentId() {
return studentId;
}
public void setStudentName(String studentName) {
this.studentName = studentName;
}
@Column(name="STUDENT_NAME", nullable=false)
public String getStudentName() {
return studentName;
}
}
Package Structure
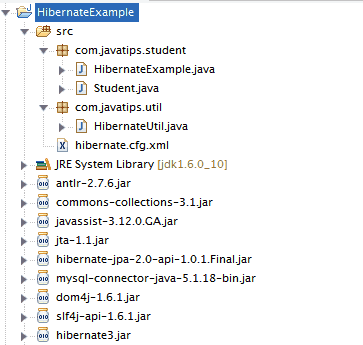
Table Structure
Consider the table with following structure
+------------+--------------+ | STUDENT_ID | STUDENT_NAME | +------------+--------------+ | 1 | Sumith | | 2 | Anoop | | 3 | Rockey | +------------+--------------+
1) HQL Select Query Example
Query query = session.createQuery("from Student where STUDENT_ID = :id ");
query.setParameter("id", "2");
List<Student> list = query.list();
query = session.createQuery("from Student where STUDENT_ID = '2' ");
list = query.list();
2) HQL Update Query Example
Query query = session.createQuery("update Student set STUDENT_NAME = :studentName" +" where STUDENT_ID = :id");
query.setParameter("id", "2");
query.setParameter("studentName", "Rose");
int result = query.executeUpdate();
query = session.createQuery("update Student set STUDENT_NAME = 'Rose'" +" where STUDENT_ID = '3'");
result = query.executeUpdate();
3) HQL Update Delete Example
Query query = session.createQuery("delete Student where STUDENT_ID = :id ");
query.setParameter("id", "2");
int result = query.executeUpdate();
query = session.createQuery("delete Student where STUDENT_ID = '1'");
result = query.executeUpdate();
Testing (HibernateExample.java)
import java.util.List;
import org.hibernate.HibernateException;
import org.hibernate.Query;
import org.hibernate.Session;
import org.hibernate.Transaction;
import com.javatips.util.HibernateUtil;
public class HibernateExample {
public static void main(String[] args) {
HibernateExample example = new HibernateExample();
example.listStudent();
example.updateStudent();
example.deleteStudent();
}
public void listStudent() {
Session session = HibernateUtil.getSessionFactory().openSession();
Transaction transaction = null;
try {
transaction = session.beginTransaction();
Query query = session.createQuery("from Student where STUDENT_ID = :id ");
query.setParameter("id", "2");
List<Student> list = query.list();
query = session.createQuery("from Student where STUDENT_ID = '2' ");
list = query.list();
transaction.commit();
} catch (HibernateException e) {
transaction.rollback();
e.printStackTrace();
} finally {
session.close();
}
}
public void updateStudent() {
Session session = HibernateUtil.getSessionFactory().openSession();
Transaction transaction = null;
try {
transaction = session.beginTransaction();
Query query = session.createQuery("update Student set STUDENT_NAME = :studentName" +" where STUDENT_ID = :id");
query.setParameter("id", "2");
query.setParameter("studentName", "Rose");
int result = query.executeUpdate();
query = session.createQuery("update Student set STUDENT_NAME = 'Rose'" +" where STUDENT_ID = '3'");
result = query.executeUpdate();
transaction.commit();
} catch (HibernateException e) {
transaction.rollback();
e.printStackTrace();
} finally {
session.close();
}
}
public void deleteStudent() {
Session session = HibernateUtil.getSessionFactory().openSession();
Transaction transaction = null;
try {
transaction = session.beginTransaction();
Query query = session.createQuery("delete Student where STUDENT_ID = :id ");
query.setParameter("id", "2");
int result = query.executeUpdate();
query = session.createQuery("delete Student where STUDENT_ID = '1'");
result = query.executeUpdate();
transaction.commit();
} catch (HibernateException e) {
transaction.rollback();
e.printStackTrace();
} finally {
session.close();
}
}
}