Java This Keyword
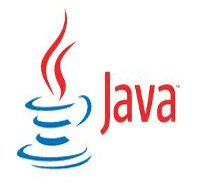
Java This Keyword Example explains step by step details of using java this keyword.
Java This keyword is a reference to the current object, it is very helpful when you need to refer an instance of a particular Object from its available methods or using it's constructor, also "this" keyword helps us to avoid naming conflicts.
The following are different ways to use java this keyword
- 1) Using with instance variable
- 2) Using with Constructor
- 3) Pass / Return current instance
Using with instance variable
Using this keyword inside a method or constructor it will use instance variable instead of local variable, in the absence of this keyword it will use local variable
public static void main(String[] args) {
Student student = new Student("Rockey", 65);
}
}
class Student {
public int marks;
public String name;
// instance variable is used when creating object
public Student(String name, int marks) {
this.name = name;
this.marks = marks;
}
}
Using with Constructor
Using this keyword inside constructor like following
this("Sony", 20);
it will call the constructor having same parameter
public static void main(String[] args) {
Student student = new Student("Rockey", 65, 22);
}
}
class Student {
public int marks;
public String name ;
public int age;
// constructor
public Student(String name, int marks) {
this.name = name;
this.marks = marks;
}
public Student(String name, int marks, int age) {
// calls another constructor
this("Sony", 20);
}
}
Pass / Return current instance
Using this keyword like following
student.calculate(this);
It will pass the current instance to appropriate method
Using this keyword with return statement like following
return this;
It will returns the current instance
public static void main(String[] args) {
Student student = new Student();
student.calculate(66, 22);
}
}
class Student {
public int marks;
public String name ;
public int age;
public Student calculate(int marks, int age) {
this.marks = marks;
this.age = age;
return this;
}
}