Hibernate Tutorial
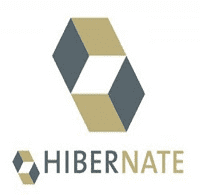
Hibernate Tutorial explains step by step details of setting / configuring Hibernate with Eclipse
Hibernate is popular open source ORM (Object Relation Mapping) tool for Java platform, for mapping an entity to a traditional relational database like Oracle, MySQL etc
By using Hibernate, we can done database operations like Create, Read, Update & Delete etc, with very little code.
We can also use Hibernate Query Language (HQL) for the jdbc operation, you can see an example of Hibernate Query Tutorial
If you want to configure Hibernate using annotations, then you can follow this example Hibernate Annotations Tutorial
Required Libraries
You need to download
Following jar must be in classpath
- antlr-2.7.6.jar
- commons-collections-3.1.jar
- javassist-3.12.0.GA.jar
- jta-1.1.jar
- hibernate-jpa-2.0-api-1.0.1.Final.jar
- hibernate3.jar
- mysql-connector-java-5.1.18-bin.jar
- dom4j-1.6.1.jar
- slf4j-api-1.6.1.jar
I am creating a sample project that persists Student object (simple POJO (Plain Old Java Object)) into database using hibernate.
Firstly create a Java Project (File->New->Project), select Java Project and click next, provide name as "HibernateExample" according to following screenshot
hibernate.cfg.xml
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name="hibernate.connection.driver_class">com.mysql.jdbc.Driver</property> <property name="hibernate.connection.url">jdbc:mysql://localhost/test</property> <property name="hibernate.connection.username">root</property> <property name="connection.password">root</property> <property name="connection.pool_size">1</property> <property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property> <property name="show_sql">true</property> <property name="hbm2ddl.auto">create</property> <mapping resource="com/javatips/student/Student.hbm.xml"/> </session-factory> </hibernate-configuration>
HibernateUtil.java
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class HibernateUtil {
private static final SessionFactory sessionFactory;
static {
try {
sessionFactory = new Configuration().configure().buildSessionFactory();
} catch (Throwable ex) {
System.err.println("Initial SessionFactory creation failed." + ex);
throw new ExceptionInInitializerError(ex);
}
}
public static SessionFactory getSessionFactory() {
return sessionFactory;
}
}
Create a Student Object(Student.java)
public class Student implements java.io.Serializable {
private long studentId;
private String studentName;
public void setStudentId(long studentId) {
this.studentId = studentId;
}
public long getStudentId() {
return studentId;
}
public void setStudentName(String studentName) {
this.studentName = studentName;
}
public String getStudentName() {
return studentName;
}
}
Student.hbm.xml
This is the hibernate mapping file used to map the domain (entity class) with database table
<?xml version="1.0"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <class name="com.javatips.student.Student" table="StudentDetails"> <meta attribute="class-description"> This class contains the student details. </meta> <id name="studentId" type="long" column="STUDENT_ID"> <generator class="native" /> </id> <property name="studentName" type="string" column="STUDENT_NAME" not-null="true" /> </class> </hibernate-mapping>
Package Structure
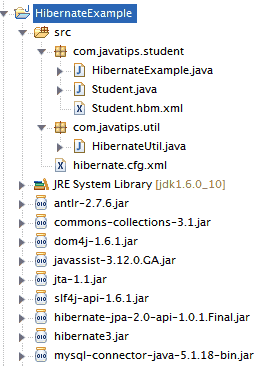
Testing (HibernateExample.java)
import java.util.List;
import java.util.Iterator;
import org.hibernate.HibernateException;
import org.hibernate.Session;
import org.hibernate.Transaction;
import com.javatips.util.HibernateUtil;
public class HibernateExample {
public static void main(String[] args) {
HibernateExample example = new HibernateExample();
Long studentId2 = example.saveStudent("Sumith");
Long studentId3 = example.saveStudent("Anoop");
example.listStudent();
example.updateStudent(studentId3, "Rockey");
example.deleteStudent(studentId2);
example.listStudent();
}
public Long saveStudent(String studentName) {
Session session = HibernateUtil.getSessionFactory().openSession();
Transaction transaction = null;
Long studentId = null;
try {
transaction = session.beginTransaction();
Student student = new Student();
student.setStudentName(studentName);
studentId = (Long) session.save(student);
transaction.commit();
} catch (HibernateException e) {
transaction.rollback();
e.printStackTrace();
} finally {
session.close();
}
return studentId;
}
public void listStudent() {
Session session = HibernateUtil.getSessionFactory().openSession();
Transaction transaction = null;
try {
transaction = session.beginTransaction();
@SuppressWarnings("unchecked")
List<Student> Students = session.createQuery("from Student").list();
for (Iterator<Student> iterator = Students.iterator(); iterator.hasNext();) {
Student student = (Student) iterator.next();
System.out.println(student.getStudentName());
}
transaction.commit();
} catch (HibernateException e) {
transaction.rollback();
e.printStackTrace();
} finally {
session.close();
}
}
public void updateStudent(Long studentId, String studentName) {
Session session = HibernateUtil.getSessionFactory().openSession();
Transaction transaction = null;
try {
transaction = session.beginTransaction();
Student student = (Student) session.get(Student.class, studentId);
student.setStudentName(studentName);
transaction.commit();
} catch (HibernateException e) {
transaction.rollback();
e.printStackTrace();
} finally {
session.close();
}
}
public void deleteStudent(Long studentId) {
Session session = HibernateUtil.getSessionFactory().openSession();
Transaction transaction = null;
try {
transaction = session.beginTransaction();
Student student = (Student) session.get(Student.class, studentId);
session.delete(student);
transaction.commit();
} catch (HibernateException e) {
transaction.rollback();
e.printStackTrace();
} finally {
session.close();
}
}
}
Output
Hibernate: insert into StudentDetails (STUDENT_NAME) values (?) Hibernate: insert into StudentDetails (STUDENT_NAME) values (?) Hibernate: select student0_.STUDENT_ID as STUDENT1_0_, student0_.STUDENT_NAME as STUDENT2_0_ from StudentDetails student0_ Sumith Anoop Hibernate: select student0_.STUDENT_ID as STUDENT1_0_0_, student0_.STUDENT_NAME as STUDENT2_0_0_ from StudentDetails student0_ where student0_.STUDENT_ID=? Hibernate: update StudentDetails set STUDENT_NAME=? where STUDENT_ID=? Hibernate: select student0_.STUDENT_ID as STUDENT1_0_0_, student0_.STUDENT_NAME as STUDENT2_0_0_ from StudentDetails student0_ where student0_.STUDENT_ID=? Hibernate: delete from StudentDetails where STUDENT_ID=? Hibernate: select student0_.STUDENT_ID as STUDENT1_0_, student0_.STUDENT_NAME as STUDENT2_0_ from StudentDetails student0_ Rockey