Create Thread In Java Web Application
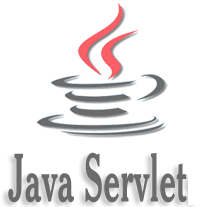
Create Thread In Java Web Application explains about implementing a background thread in a java web application so that you can do some logic behind these background thread.
You can achieve thread implementation in j2ee web application several ways. For example if you are using application servers like Jboss,Weblogic & Websphere, you have more possible ways to do this. But here we are targeting for both servlet containers like Tomcat, jetty etc and application servers like JBOSS, Weblogic etc.
We are showing implementing Thread in Java web application two different ways:
1) Create Thread Using ServletContextListener 2) Create Thread Using HttpServlet
Required Libraries
You need to download
1) Create Thread Using ServletContextListener
For the entire web application, there will be only one ServletContext, so ServletContextListner notified when any change happens to the ServletContext lifecycle
You can see more about implementing interface ServletContextListener ServletContextListener Example here.
Here we are using ScheduledExecutorService when contextInitialized method is invoked , we are printing the current date at periodic intervals, you can change the logic inside run method as per your requirements. After doing the tasks, you can able to clean up the thread (shutdown) when contextDestroyed method is invoked.
More clarification, please check the below code
ThreadListenerExample.java
import java.util.Date;
import java.util.TimerTask;
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
import javax.servlet.ServletContextEvent;
import javax.servlet.ServletContextListener;
public class ThreadListenerExample implements ServletContextListener {
private ScheduledExecutorService scheduler = null;
public void contextInitialized(ServletContextEvent sce) {
if ((scheduler == null) || (!scheduler.isTerminated())) {
scheduler = Executors.newSingleThreadScheduledExecutor();
scheduler.scheduleAtFixedRate(new ScheduledTask(), 0, 3, TimeUnit.SECONDS);
}
}
public void contextDestroyed(ServletContextEvent sce) {
try {
System.out.println("Scheduler Shutting down successfully " + new Date());
scheduler.shutdown();
} catch (Exception ex) {
}
}
}
class ScheduledTask extends TimerTask {
public void run() {
System.out.println(new Date());
}
}
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" version="2.5"> <listener> <listener-class>com.listener.ThreadListenerExample</listener-class> </listener> </web-app>
Package Structure
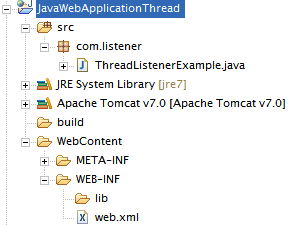
Output
Fri Apr 29 08:13:51 IST 2014 Fri Apr 29 08:13:54 IST 2014 Fri Apr 29 08:13:57 IST 2014 Fri Apr 29 08:14:00 IST 2014 Fri Apr 29 08:14:03 IST 2014 Fri Apr 29 08:14:06 IST 2014 Fri Apr 29 08:14:09 IST 2014 Fri Apr 29 08:14:12 IST 2014 Fri Apr 29 08:14:15 IST 2014 Fri Apr 29 08:14:18 IST 2014 Fri Apr 29 08:14:21 IST 2014 Fri Apr 29 08:14:24 IST 2014 Fri Apr 29 08:14:27 IST 2014 Fri Apr 29 08:14:30 IST 2014 Fri Apr 29 08:14:33 IST 2014 Fri Apr 29 08:14:36 IST 2014 Fri Apr 29 08:14:39 IST 2014 Fri Apr 29 08:14:42 IST 2014 ............................ ............................ ............................
2) Create Thread Using HttpServlet
Here we are using Thread in java when init() method of HttpServlet invoked , we are printing the current date at periodic intervals, you can change the logic inside run method as per your requirements.
Also see that we are used <load-on-startup> inside web.xml, this is for preloading the servlet
More clarification, please check the below code
ThreadServletExample.java
import java.util.Date;
import javax.servlet.http.HttpServlet;
public class ThreadServletExample extends HttpServlet implements Runnable {
private static final long serialVersionUID = 1L;
public void init() {
try {
Thread thread = new Thread(this);
thread.start();
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
public void run() {
while (true) {
try {
System.out.println(new Date());
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" version="2.5"> <servlet> <servlet-name>ThreadServletExample</servlet-name> <servlet-class>com.thread.ThreadServletExample</servlet-class> <load-on-startup>1</load-on-startup> </servlet> </web-app>
Package Structure
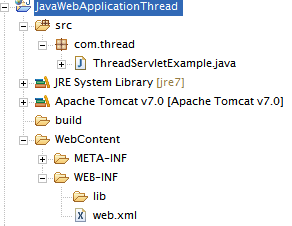
Output
Fri Apr 29 08:13:51 IST 2014 Fri Apr 29 08:13:54 IST 2014 Fri Apr 29 08:13:57 IST 2014 Fri Apr 29 08:14:00 IST 2014 Fri Apr 29 08:14:03 IST 2014 Fri Apr 29 08:14:06 IST 2014 Fri Apr 29 08:14:09 IST 2014 Fri Apr 29 08:14:12 IST 2014 Fri Apr 29 08:14:15 IST 2014 Fri Apr 29 08:14:18 IST 2014 Fri Apr 29 08:14:21 IST 2014 Fri Apr 29 08:14:24 IST 2014 Fri Apr 29 08:14:27 IST 2014 Fri Apr 29 08:14:30 IST 2014 Fri Apr 29 08:14:33 IST 2014 Fri Apr 29 08:14:36 IST 2014 Fri Apr 29 08:14:39 IST 2014 Fri Apr 29 08:14:42 IST 2014 ............................ ............................ ............................